在Spring的配置文件中,配置切面使用的是____元素
时间: 2024-10-20 14:06:42 浏览: 24
在Spring的配置文件中,用于配置切面(AspectJ或Spring AOP)的元素是`<aop:config>`。这个元素包含了一系列的子标签,如 `<aop:aspect>`(表示一个切面)、`<aop:before>`, `<aop:after>` 等,用来声明通知(Advice)和切入点(Pointcut Expression)等切面的相关信息。通过这种方式,可以在XML配置中声明切面如何拦截目标类的行为,并注入自定义的逻辑。
例如:
```xml
<aop:config>
<aop:aspect id="myAspect">
<aop:before method="beforeMethod" pointcut="execution(* com.example.service.*.*(..))"/>
<!-- 其他通知 -->
</aop:aspect>
</aop:config>
```
在这里,`myAspect`是一个切面,`beforeMethod`是一个前置通知,它会在方法执行前被调用,而`execution(* com.example.service.*.*(..))`是一个表达式,定义了切点,即被拦截的目标方法集合。
相关问题
Spring配置文件是什么
Spring配置文件是用来配置Spring应用程序的XML文件。它包含了Spring的各种配置元素,如bean定义、依赖注入、AOP配置、数据源配置等。Spring提供了多种配置方式,包括XML配置、注解配置和Java配置。XML配置是最常用的一种方式,它使用XML文档来描述应用程序的配置信息。在XML配置文件中,可以定义bean的属性、依赖、作用域等信息,以及AOP切面、事务管理等高级配置信息。通过读取Spring配置文件,Spring容器可以创建对象、注入依赖、管理生命周期等。
springaop配置文件
Spring AOP配置文件主要有两种方式:基于XML配置和基于注解配置。
基于XML配置的方式需要在Spring配置文件中引入aop的命名空间,并通过<aop:config>元素来定义切面和通知。具体配置如下:
1. 引入aop的命名空间:
```xml
xmlns:aop="http://www.springframework.org/schema/aop"
```
2. 在<aop:config>中定义切面和通知:
```xml
<aop:config>
<aop:aspect id="myAspect" ref="myAspectBean">
<aop:pointcut expression="execution(* com.example.MyClass.myMethod(..))" id="myPointcut" />
<aop:before method="beforeAdvice" pointcut-ref="myPointcut" />
<aop:after method="afterAdvice" pointcut-ref="myPointcut" />
</aop:aspect>
</aop:config>
```
其中,id为"myAspect"的元素定义了切面,ref属性指定了切面所对应的Bean。expression属性指定了切点表达式,pointcut-ref属性指定了切点,method属性指定了通知方法。
基于注解配置的方式需要在Spring配置文件中开启注解支持,并在切面类上使用相应的注解进行配置。具体配置如下:
1. 开启注解支持:
```xml
<context:annotation-config />
<aop:aspectj-autoproxy />
```
2. 在切面类上使用注解进行配置:
```java
@Aspect
@Component
public class MyAspect {
@Pointcut("execution(* com.example.MyClass.myMethod(..))")
public void myPointcut() {}
@Before("myPointcut()")
public void beforeAdvice() {
// 前置通知的逻辑
}
@After("myPointcut()")
public void afterAdvice() {
// 后置通知的逻辑
}
}
```
其中,@Aspect注解标识该类为切面,@Component注解将该类作为Spring的Bean进行管理。@Pointcut注解定义了切点,@Before注解定义了前置通知方法,@After注解定义了后置通知方法。
阅读全文
相关推荐
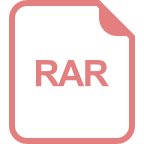
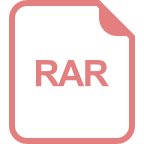
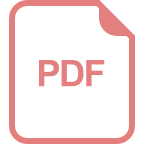
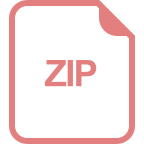
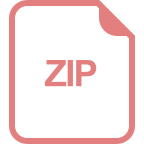
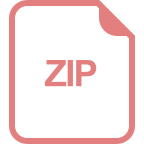
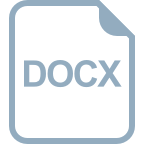
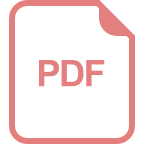
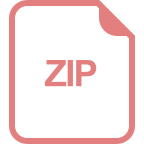
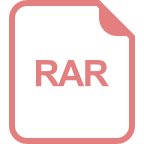
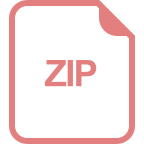
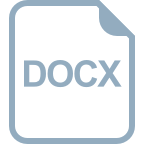
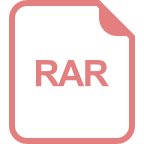
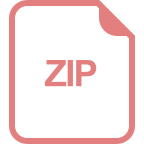
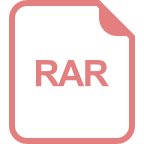
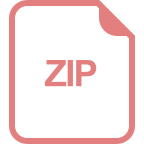