帮我找个金融知识库系统的代码
时间: 2023-06-24 07:03:33 浏览: 55
金融知识库系统的代码实现需要考虑很多方面,比如后端数据库的设计、前端页面的开发、用户权限管理等。这里提供一个简单的参考代码,供你参考:
1. 数据库设计
首先,需要设计数据库来存储金融知识库的内容。以下是一个简单的示例:
```sql
CREATE TABLE article (
id INT AUTO_INCREMENT PRIMARY KEY,
title VARCHAR(255) NOT NULL,
content TEXT NOT NULL,
author VARCHAR(50) NOT NULL,
create_time DATETIME NOT NULL
);
CREATE TABLE category (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(50) NOT NULL
);
CREATE TABLE article_category (
article_id INT NOT NULL,
category_id INT NOT NULL,
PRIMARY KEY (article_id, category_id),
FOREIGN KEY (article_id) REFERENCES article(id),
FOREIGN KEY (category_id) REFERENCES category(id)
);
```
2. 后端代码
使用 Python 的 Flask 框架来实现后端代码。以下是一个简单的示例:
```python
from flask import Flask, request, jsonify
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'mysql://user:password@localhost/dbname'
db = SQLAlchemy(app)
class Article(db.Model):
id = db.Column(db.Integer, primary_key=True)
title = db.Column(db.String(255), nullable=False)
content = db.Column(db.Text, nullable=False)
author = db.Column(db.String(50), nullable=False)
create_time = db.Column(db.DateTime, nullable=False)
class Category(db.Model):
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(50), nullable=False)
class ArticleCategory(db.Model):
article_id = db.Column(db.Integer, db.ForeignKey('article.id'), primary_key=True)
category_id = db.Column(db.Integer, db.ForeignKey('category.id'), primary_key=True)
@app.route('/articles', methods=['GET'])
def get_articles():
articles = Article.query.all()
return jsonify([article.serialize() for article in articles])
@app.route('/articles', methods=['POST'])
def create_article():
data = request.get_json()
article = Article(title=data['title'], content=data['content'], author=data['author'])
db.session.add(article)
db.session.commit()
return jsonify(article.serialize())
@app.route('/articles/<int:id>', methods=['GET'])
def get_article(id):
article = Article.query.get(id)
return jsonify(article.serialize())
@app.route('/articles/<int:id>', methods=['PUT'])
def update_article(id):
data = request.get_json()
article = Article.query.get(id)
article.title = data['title']
article.content = data['content']
article.author = data['author']
db.session.commit()
return jsonify(article.serialize())
@app.route('/articles/<int:id>', methods=['DELETE'])
def delete_article(id):
article = Article.query.get(id)
db.session.delete(article)
db.session.commit()
return jsonify({'message': 'Article deleted'})
if __name__ == '__main__':
app.run(debug=True)
```
3. 前端代码
使用 React 框架来实现前端页面。以下是一个简单的示例:
```jsx
import React, { useState, useEffect } from 'react';
import axios from 'axios';
function App() {
const [articles, setArticles] = useState([]);
const [title, setTitle] = useState('');
const [content, setContent] = useState('');
const [author, setAuthor] = useState('');
useEffect(() => {
axios.get('/articles')
.then(response => setArticles(response.data))
.catch(error => console.log(error));
}, []);
const handleSubmit = event => {
event.preventDefault();
axios.post('/articles', { title, content, author })
.then(response => setArticles([...articles, response.data]))
.catch(error => console.log(error));
setTitle('');
setContent('');
setAuthor('');
};
const handleDelete = id => {
axios.delete(`/articles/${id}`)
.then(() => setArticles(articles.filter(article => article.id !== id)))
.catch(error => console.log(error));
};
return (
<div>
<h1>金融知识库</h1>
<form onSubmit={handleSubmit}>
<input type="text" value={title} onChange={event => setTitle(event.target.value)} placeholder="标题" />
<textarea value={content} onChange={event => setContent(event.target.value)} placeholder="内容"></textarea>
<input type="text" value={author} onChange={event => setAuthor(event.target.value)} placeholder="作者" />
<button type="submit">提交</button>
</form>
<ul>
{articles.map(article => (
<li key={article.id}>
<h2>{article.title}</h2>
<p>{article.content}</p>
<small>{article.author} / {article.create_time}</small>
<button onClick={() => handleDelete(article.id)}>删除</button>
</li>
))}
</ul>
</div>
);
}
export default App;
```
以上是一个简单的金融知识库系统的代码实现,供你参考。当然,实际开发中还需要考虑很多细节问题,比如用户权限管理、搜索功能、分类功能等等。
相关推荐
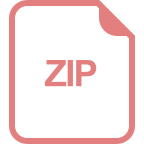
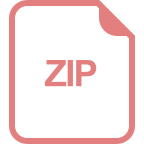
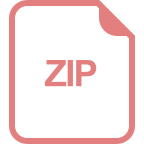














