用c++编写一个停车管理系统
时间: 2023-08-04 22:13:57 浏览: 92
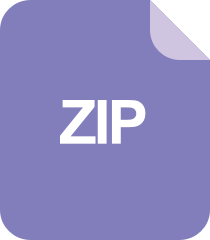
C++编写的停车场管理系统
以下是一个简单的停车管理系统的C++代码示例:
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class ParkingLot {
private:
int capacity;
vector<string> parkedCars;
public:
ParkingLot(int capacity) {
this->capacity = capacity;
}
bool isFull() {
return parkedCars.size() == capacity;
}
void parkCar(string licensePlate) {
if (isFull()) {
cout << "Sorry, the parking lot is full." << endl;
} else {
parkedCars.push_back(licensePlate);
cout << "Car with license plate " << licensePlate << " has been parked." << endl;
}
}
void unparkCar(string licensePlate) {
bool found = false;
for (int i = 0; i < parkedCars.size(); i++) {
if (parkedCars[i] == licensePlate) {
parkedCars.erase(parkedCars.begin() + i);
cout << "Car with license plate " << licensePlate << " has been unparked." << endl;
found = true;
break;
}
}
if (!found) {
cout << "Car with license plate " << licensePlate << " is not parked here." << endl;
}
}
void printStatus() {
cout << "Parking lot has " << capacity - parkedCars.size() << " empty spots." << endl;
cout << "Parked cars:" << endl;
for (string licensePlate : parkedCars) {
cout << "- " << licensePlate << endl;
}
}
};
int main() {
ParkingLot parkingLot(5);
parkingLot.parkCar("ABC-123");
parkingLot.parkCar("DEF-456");
parkingLot.parkCar("GHI-789");
parkingLot.printStatus();
parkingLot.unparkCar("DEF-456");
parkingLot.printStatus();
parkingLot.parkCar("JKL-012");
parkingLot.parkCar("MNO-345");
parkingLot.parkCar("PQR-678");
parkingLot.printStatus();
parkingLot.parkCar("STU-901");
return 0;
}
```
此代码中,`ParkingLot`类代表了一个停车场,它有一个最大容量和一个记录当前停放车辆的`vector`。`isFull()`函数检查停车场是否已满,`parkCar()`函数将车辆停入停车场,`unparkCar()`函数将车辆从停车场取出,`printStatus()`函数列出当前停放车辆和剩余停车位数量。
在`main()`函数中,我们创建了一个容量为5的停车场,并进行了一些停放和取出车辆的操作,最后列出了当前停放车辆和剩余停车位数量的信息。
阅读全文
相关推荐
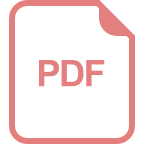
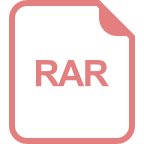
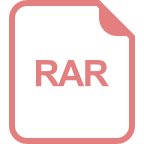
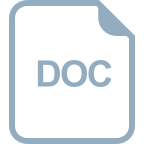
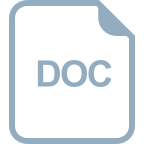
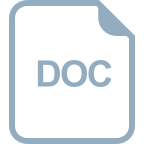


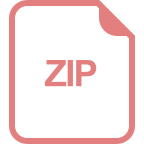
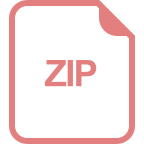
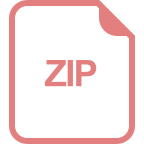
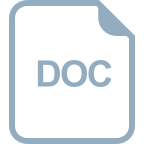
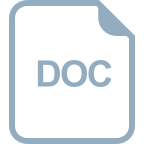
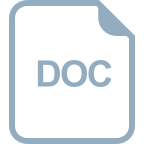
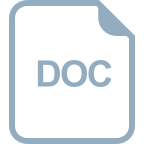