基于cnn的文本分类的pytorch实现
时间: 2023-05-14 10:01:46 浏览: 175
基于cnn的文本分类是一种基于神经网络的文本分类方法,其主要特点是使用卷积神经网络(CNN)对文本特征进行提取和表示,进而实现文本分类。PyTorch是一种基于Python的深度学习框架,自2016年发布以来逐渐成为深度学习领域的热门工具之一。本文将介绍如何使用PyTorch实现基于CNN的文本分类。
在PyTorch中实现基于CNN的文本分类,需要根据以下步骤进行:
1. 数据预处理:包括数据清洗、分词、构建词汇表等。
2. 构建模型:使用PyTorch搭建CNN模型,包括卷积层、池化层和全连接层等。
3. 模型训练:使用训练集对模型进行训练,包括确定超参数和选择优化器等。
4. 模型评估:使用验证集对模型进行评估,包括计算准确率、召回率、F1值等。
5. 模型预测:使用测试集对模型进行预测,得出最终分类结果。
在具体实现时,可以使用PyTorch提供的各种库和函数,如torch.nn、torch.utils.data和torch.optim等。通过调试和优化模型,可以得到较好的分类效果。
基于CNN的文本分类具有较高的准确率和泛化能力,在实际应用中具有广泛的应用前景。 PyTorch作为一种实用性强、易于学习和使用的深度学习框架,可以为基于CNN的文本分类提供强有力的支持和便捷的实现方式。
相关推荐
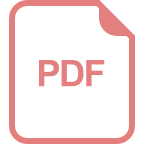
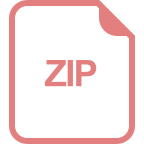
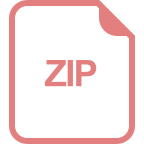
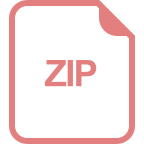
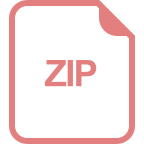
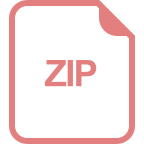
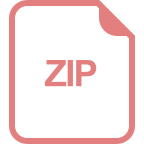
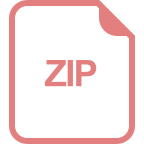
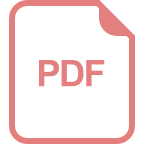
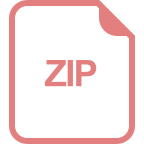