Create a Single Page Application (SPA) in React App with proper navigation.
时间: 2024-03-17 17:39:39 浏览: 80
To create a SPA in React, we can use React Router. Here's an example of how to create a SPA in React with proper navigation:
1. First, create a new React app using `create-react-app`.
2. Install React Router by running the following command in your terminal:
```
npm install react-router-dom
```
3. In your `src` folder, create a new folder called `pages`. Inside this folder, create a new file for each of your pages. For example, you could create a `Home.js` file and a `About.js` file.
4. In each of your page files, create a React component that represents that page. For example:
```jsx
import React from 'react';
function Home() {
return (
<div>
<h1>Welcome to my website!</h1>
<p>Here is some content.</p>
</div>
);
}
export default Home;
```
5. In your `App.js` file, import `BrowserRouter` from `react-router-dom` and wrap your entire app in it. Then, create a `Switch` component that will render the appropriate page based on the URL.
```jsx
import React from 'react';
import { BrowserRouter, Switch, Route, Link } from 'react-router-dom';
import Home from './pages/Home';
import About from './pages/About';
function App() {
return (
<BrowserRouter>
<nav>
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
</ul>
</nav>
<Switch>
<Route path="/about">
<About />
</Route>
<Route path="/">
<Home />
</Route>
</Switch>
</BrowserRouter>
);
}
export default App;
```
6. In the example above, we're using `Link` components to create links to our pages, and we're using `Route` components to specify which component should be rendered for each URL. The `Switch` component ensures that only one page is rendered at a time.
7. Start your app by running `npm start` in your terminal. You should see your navigation links and be able to navigate between your pages.
阅读全文
相关推荐
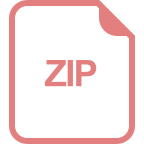
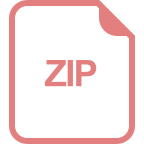
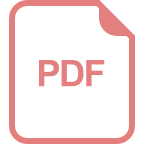
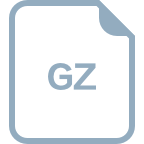
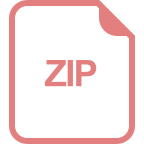
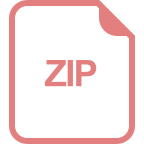
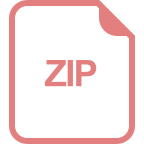
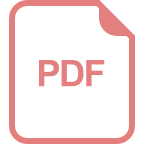
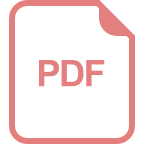
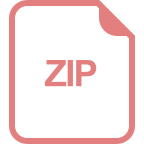
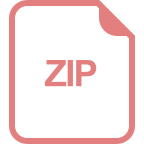
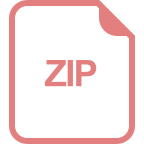
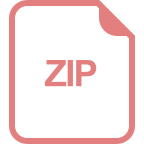
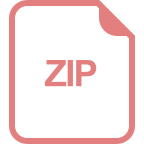
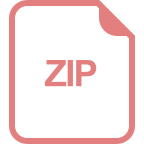
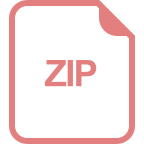
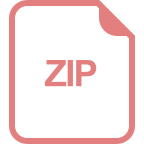
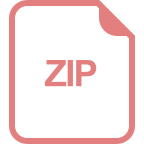
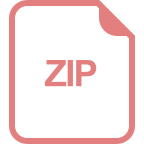