UnboundLocalError: cannot access local variable 'judge_a' where it is not associated with a value
时间: 2024-02-21 16:01:21 浏览: 94
This error occurs when you try to access a local variable that has not been assigned a value within the current function.
To fix this error, you need to ensure that the variable is assigned a value before you try to use it. You can do this by initializing the variable before using it, or by passing in a value as an argument when calling the function.
For example:
```
def my_function():
judge_a = None # initialize the variable
# rest of the code
my_function() # call the function
```
Or:
```
def my_function(judge_a):
# rest of the code
my_function(None) # call the function with a default value
```
相关问题
UnboundLocalError: cannot access local variable 'a' where it is not associated with a value
这个错误通常是因为在函数内部使用变量时,该变量没有被正确地赋值。请确保在使用变量之前为其分配一个值。例如:
```
def my_function():
a = 10
print(a)
my_function()
```
在这个例子中,变量 `a` 被分配了一个值 `10`,然后在函数内部使用并打印出来。如果 `a` 没有在函数内部分配一个值,就会出现 "UnboundLocalError: cannot access local variable 'a' where it is not associated with a value" 错误。
UnboundLocalError: cannot access local variable 'sslerror' where it is not associated with a value
这个错误是因为你在函数内部使用了一个未定义的本地变量,而且在该函数内部也没有给该变量赋值。在 Python 中,只有在函数内部显式地声明一个变量为全局变量时,才能在函数中访问该变量。如果你在函数中使用一个变量,但是没有给它赋值,那么 Python 就会认为这个变量是一个本地变量,因此当你尝试访问它时,就会出现 UnboundLocalError 错误。
要解决这个错误,你需要检查你的代码,并确保在使用变量之前,它已经被正确地定义和赋值了。如果你需要在函数内部使用一个全局变量,你可以使用 global 关键字来声明它。例如:
```
def my_function():
global my_variable
my_variable = 42
```
这样就可以在函数内部访问和修改全局变量 my_variable 了。但是在使用全局变量时,要注意避免命名冲突和意外修改全局变量的值。
相关推荐
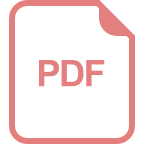
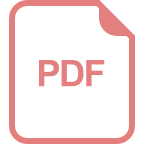
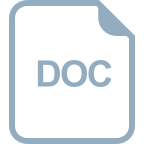












