SpringBoot整合Curator
时间: 2024-01-18 13:02:00 浏览: 95
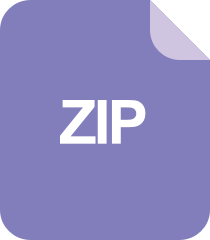
zookeeper-console:基于SpringBoot,Curator和Bootstrap的Zookeeper可视化Web应用程序
Curator是一个ZooKeeper客户端库,用于简化ZooKeeper API的使用。Spring Boot是一个非常流行的Java应用程序框架。将它们结合起来,可以使开发人员更轻松地使用ZooKeeper来实现分布式应用程序。
以下是Spring Boot整合Curator的步骤:
1. 添加Curator依赖
在`pom.xml`文件中添加以下依赖:
```xml
<dependency>
<groupId>org.apache.curator</groupId>
<artifactId>curator-framework</artifactId>
<version>4.2.0</version>
</dependency>
<dependency>
<groupId>org.apache.curator</groupId>
<artifactId>curator-recipes</artifactId>
<version>4.2.0</version>
</dependency>
```
2. 配置ZooKeeper连接
在`application.yml`文件中添加以下配置:
```yaml
zookeeper:
connectString: 127.0.0.1:2181
namespace: myapp
```
3. 创建Curator客户端
在Spring Boot应用程序中创建Curator客户端,例如:
```java
@Configuration
public class ZookeeperConfig {
@Autowired
private ZookeeperProperties properties;
@Bean
public CuratorFramework curatorFramework() {
String connectString = properties.getConnectString();
String namespace = properties.getNamespace();
CuratorFramework curatorFramework = CuratorFrameworkFactory.builder()
.connectString(connectString)
.namespace(namespace)
.retryPolicy(new ExponentialBackoffRetry(1000, 3))
.build();
curatorFramework.start();
return curatorFramework;
}
}
```
4. 使用Curator客户端
使用Curator客户端实现ZooKeeper的常用操作,例如:
```java
@Autowired
private CuratorFramework curatorFramework;
public void createNode(String path, byte[] data) throws Exception {
curatorFramework.create().creatingParentsIfNeeded().forPath(path, data);
}
public void deleteNode(String path) throws Exception {
curatorFramework.delete().deletingChildrenIfNeeded().forPath(path);
}
public byte[] getData(String path) throws Exception {
return curatorFramework.getData().forPath(path);
}
public void setData(String path, byte[] data) throws Exception {
curatorFramework.setData().forPath(path, data);
}
public List<String> getChildren(String path) throws Exception {
return curatorFramework.getChildren().forPath(path);
}
```
这些步骤可以让你方便地使用Curator作为ZooKeeper客户端库来实现分布式应用程序。
阅读全文
相关推荐
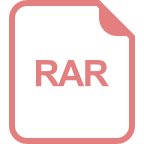
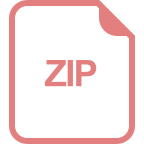









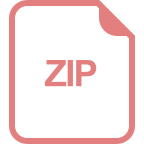
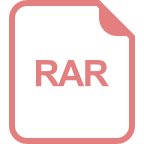
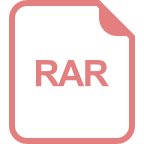
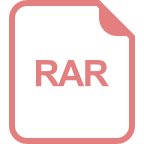
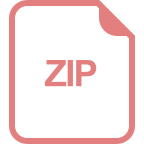