基于boost1.70,UDP通讯,实现一个客户端和服务器交互,举个例子
时间: 2024-03-18 15:40:18 浏览: 116
好的,我可以给您举一个基于Boost 1.70和UDP通信实现客户端和服务器交互的例子。
首先,我们来看客户端的实现。客户端需要创建一个UDP socket对象,并绑定到本地的IP地址和端口号。然后,通过`socket.send_to()`函数向服务器发送数据,最后通过`socket.receive_from()`函数从服务器接收数据。具体代码如下:
```cpp
#include <iostream>
#include <boost/asio.hpp>
int main()
{
try
{
boost::asio::io_context io_context;
boost::asio::ip::udp::socket socket(io_context);
// 绑定到本地的IP地址和端口号
boost::asio::ip::udp::endpoint local_endpoint(boost::asio::ip::address::from_string("127.0.0.1"), 8888);
socket.open(local_endpoint.protocol());
socket.bind(local_endpoint);
// 发送数据到服务器
boost::asio::ip::udp::endpoint server_endpoint(boost::asio::ip::address::from_string("127.0.0.1"), 9999);
std::string message = "Hello, server!";
socket.send_to(boost::asio::buffer(message), server_endpoint);
// 接收服务器返回的数据
char recv_buffer[1024];
boost::asio::ip::udp::endpoint remote_endpoint;
size_t len = socket.receive_from(boost::asio::buffer(recv_buffer), remote_endpoint);
std::cout << "Received from server: " << std::string(recv_buffer, len) << std::endl;
}
catch (std::exception& e)
{
std::cerr << "Exception: " << e.what() << std::endl;
}
return 0;
}
```
接下来,我们来看服务器的实现。服务器需要创建一个UDP socket对象,并绑定到本地的IP地址和端口号。然后,通过`socket.receive_from()`函数从客户端接收数据,最后通过`socket.send_to()`函数向客户端发送数据。具体代码如下:
```cpp
#include <iostream>
#include <boost/asio.hpp>
int main()
{
try
{
boost::asio::io_context io_context;
boost::asio::ip::udp::socket socket(io_context);
// 绑定到本地的IP地址和端口号
boost::asio::ip::udp::endpoint local_endpoint(boost::asio::ip::address::from_string("127.0.0.1"), 9999);
socket.open(local_endpoint.protocol());
socket.bind(local_endpoint);
// 接收客户端发送的数据
char recv_buffer[1024];
boost::asio::ip::udp::endpoint remote_endpoint;
size_t len = socket.receive_from(boost::asio::buffer(recv_buffer), remote_endpoint);
std::cout << "Received from client: " << std::string(recv_buffer, len) << std::endl;
// 发送数据到客户端
std::string message = "Hello, client!";
socket.send_to(boost::asio::buffer(message), remote_endpoint);
}
catch (std::exception& e)
{
std::cerr << "Exception: " << e.what() << std::endl;
}
return 0;
}
```
以上就是一个基于Boost 1.70和UDP通信实现客户端和服务器交互的例子,您可以根据自己的需求进行适当的修改。
阅读全文
相关推荐
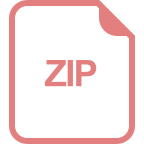
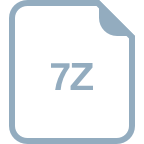
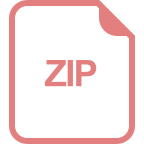
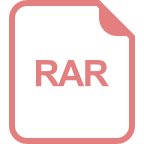
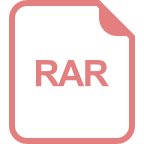
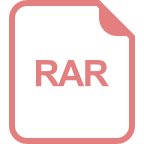
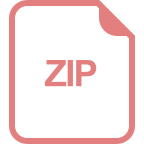
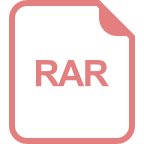
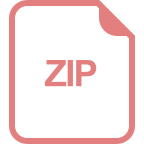
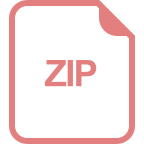
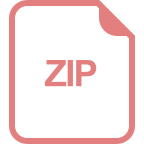
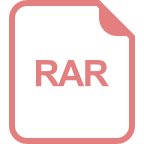
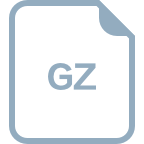
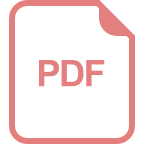
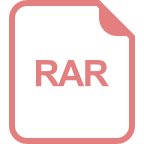