springboot实现HealthIndicator
时间: 2023-09-20 22:05:47 浏览: 159
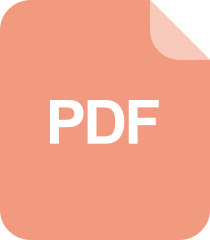
spring boot starter actuator(健康监控)配置和使用教程
### 回答1:
在Spring Boot中,我们可以通过实现HealthIndicator接口来实现自定义健康检查。
步骤如下:
1. 定义一个实现了HealthIndicator接口的类。
```java
@Component
public class MyHealthIndicator implements HealthIndicator {
@Override
public Health health() {
// 自定义健康检查的逻辑
if (checkHealth()) {
return Health.up().build();
} else {
return Health.down().withDetail("error", "error message").build();
}
}
private boolean checkHealth() {
// 检查应用的健康状态
return true;
}
}
```
2. 在MyHealthIndicator类上添加@Component注解,将它声明为Spring Bean。
3. 启动应用程序并访问http://localhost:8080/actuator/health,就可以看到自定义的健康检查结果了。
如果健康检查的状态正常,返回结果如下:
```json
{
"status": "UP"
}
```
如果健康检查的状态不正常,返回结果如下:
```json
{
"status": "DOWN",
"details": {
"error": "error message"
}
}
```
这里需要注意的是,在返回DOWN状态时,我们可以通过withDetail方法设置错误信息。在实际应用中,我们可以根据具体的业务逻辑来设置错误信息。
另外,需要注意的是,在Spring Boot 2.x中,Actuator默认开启了安全认证,因此我们需要在application.properties中添加以下配置:
```properties
management.endpoint.health.show-details=always
```
这样才能在返回的JSON结果中看到错误信息。
### 回答2:
在Spring Boot中,HealthIndicator是一个接口,用于提供应用程序的健康状态。通过实现该接口,我们可以返回应用程序各个组件的健康状态,从而监控和管理应用程序的运行状况。
首先,我们需要创建一个自定义的HealthIndicator实现类。该类需要实现HealthIndicator接口,并重写其中的health()方法。在health()方法中,我们可以检查应用程序的各个组件,如数据库连接、缓存、第三方服务等的状况,并返回对应的结果。
例如,我们可以通过调用数据库的连接方法来判断数据库是否可用,并根据结果返回UP(可用)或者DOWN(不可用)。同样地,我们可以通过调用缓存的方法来判断缓存是否可用,并返回对应的结果。
在实现HealthIndicator的类中,我们还可以自定义一些额外的信息,例如版本号、构建时间等,这些信息将作为健康检查的一部分返回。
为了在应用程序中使用自定义的HealthIndicator,我们需要将其注册到Spring容器中。可以通过创建一个配置类,并在该类中使用@Bean将自定义的HealthIndicator实例化,并添加到HealthIndicatorRegistry中。
最后,我们可以通过访问"/actuator/health"端点来获取应用程序的健康状态。在返回的JSON中,我们可以看到每个注册的HealthIndicator的健康状态以及其他自定义的信息。
总结来说,通过实现HealthIndicator接口,我们可以在Spring Boot中实现应用程序的健康检查。这对于监控和管理应用程序的健康状态非常有帮助,能够及时发现和解决问题,保证应用程序的稳定运行。
### 回答3:
Spring Boot提供了一个插件化的扩展机制来实现HealthIndicator接口,以便我们可以根据自己的需求来定制系统的健康检查机制。
实现HealthIndicator主要需要完成以下几个步骤:
1. 创建一个用于检查系统健康的类,该类实现HealthIndicator接口,并重写health()方法。
示例代码如下:
```java
@Component
public class CustomHealthIndicator implements HealthIndicator {
@Override
public Health health() {
// 根据实际需求进行系统健康检查,并返回相应的健康状态
if (checkSystemHealth()) {
return Health.up().build();
} else {
return Health.down().withDetail("Error", "System is not healthy").build();
}
}
private boolean checkSystemHealth() {
// 实际的系统健康检查逻辑
// 返回true表示系统健康,返回false表示系统不健康
...
}
}
```
2. 注册CustomHealthIndicator到Spring Boot中。
可以通过在配置类上使用@EnableScheduling注解来启用定时调度的功能,然后使用@Scheduled注解来定期执行健康检查。
示例代码如下:
```java
@Configuration
@EnableScheduling
public class HealthIndicatorConfig {
@Autowired
private CustomHealthIndicator customHealthIndicator;
@Scheduled(cron = "0 0/5 * * * ?") // 每5分钟执行一次健康检查
public void healthCheck() {
customHealthIndicator.health();
}
}
```
通过以上的步骤,我们就可以实现自己的HealthIndicator并注册到Spring Boot中进行系统健康检查了。掌握了这个功能,我们可以根据实际需求来检查系统的健康状态,并根据健康状态来进行相应的处理。
阅读全文
相关推荐
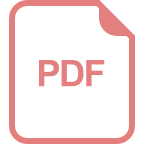
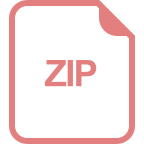
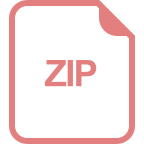
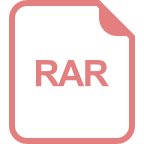
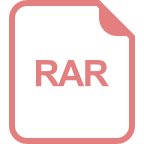
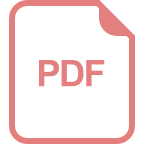
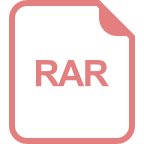
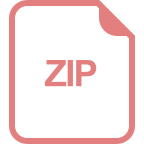
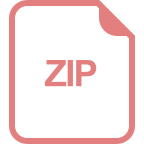
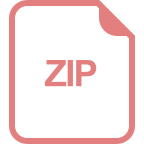
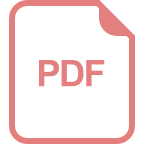
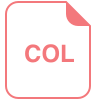




