javaee,使用JSP+servlet+Mybatis框架技术,有注册登录,产品信息增删改查
时间: 2024-03-19 17:45:30 浏览: 84
好的,以下是一个可能的实现思路和代码示例。请注意,这只是一个粗略的示例,您需要根据具体需求进行修改和优化。
1. 框架搭建
首先,您需要搭建JSP+servlet+Mybatis框架,这里提供一个简单的搭建步骤:
1.1 安装Tomcat服务器和MySQL数据库,并启动Tomcat服务器和MySQL数据库。
1.2 在Eclipse等IDE中新建一个Dynamic Web Project项目,选择Tomcat服务器。
1.3 在项目中添加必要的JSP、servlet和Mybatis等框架的jar包。
1.4 编写web.xml文件,配置servlet和servlet-mapping。
2. 管理员注册登录功能
2.1 创建管理员表
首先,您需要创建一个管理员表,用于存储管理员的信息。可以使用以下语句在MySQL数据库中创建一个管理员表:
```sql
CREATE TABLE `admin` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`username` varchar(50) NOT NULL,
`password` varchar(50) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
```
2.2 注册功能实现
在注册页面中,用户需要输入用户名和密码,然后点击注册按钮。在后台,可以使用servlet接收表单数据,然后使用Mybatis框架将数据插入到数据库中。
```java
public class RegisterServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
private AdminDao adminDao = new AdminDaoImpl();
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String username = request.getParameter("username");
String password = request.getParameter("password");
Admin admin = new Admin();
admin.setUsername(username);
admin.setPassword(password);
boolean result = adminDao.addAdmin(admin);
if(result) {
response.sendRedirect("login.jsp");
} else {
response.sendRedirect("register.jsp");
}
}
}
```
2.3 登录功能实现
在登录页面中,用户需要输入用户名和密码,然后点击登录按钮。在后台,可以使用servlet接收表单数据,然后使用Mybatis框架查询数据库中是否存在对应的管理员信息。
```java
public class LoginServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
private AdminDao adminDao = new AdminDaoImpl();
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String username = request.getParameter("username");
String password = request.getParameter("password");
Admin admin = adminDao.getAdminByUsername(username);
if(admin != null && admin.getPassword().equals(password)) {
HttpSession session = request.getSession();
session.setAttribute("admin", admin);
response.sendRedirect("index.jsp");
} else {
response.sendRedirect("login.jsp");
}
}
}
```
3. 产品信息增删改查
3.1 创建产品表
首先,您需要创建一个产品表,用于存储产品的信息。可以使用以下语句在MySQL数据库中创建一个产品表:
```sql
CREATE TABLE `product` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(50) NOT NULL,
`price` double NOT NULL,
`description` varchar(100) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
```
3.2 增加产品信息
在增加产品信息页面中,用户需要输入产品名称、价格和描述,然后点击提交按钮。在后台,可以使用servlet接收表单数据,然后使用Mybatis框架将数据插入到数据库中。
```java
public class AddProductServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
private ProductDao productDao = new ProductDaoImpl();
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String name = request.getParameter("name");
double price = Double.parseDouble(request.getParameter("price"));
String description = request.getParameter("description");
Product product = new Product();
product.setName(name);
product.setPrice(price);
product.setDescription(description);
boolean result = productDao.addProduct(product);
if(result) {
response.sendRedirect("productList.jsp");
} else {
response.sendRedirect("addProduct.jsp");
}
}
}
```
3.3 删除产品信息
在删除产品信息页面中,用户需要选择需要删除的产品,然后点击删除按钮。在后台,可以使用servlet接收表单数据,然后使用Mybatis框架从数据库中删除对应的产品信息。
```java
public class DeleteProductServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
private ProductDao productDao = new ProductDaoImpl();
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int id = Integer.parseInt(request.getParameter("id"));
boolean result = productDao.deleteProductById(id);
if(result) {
response.sendRedirect("productList.jsp");
} else {
response.sendRedirect("productList.jsp");
}
}
}
```
3.4 修改产品信息
在修改产品信息页面中,用户需要选择需要修改的产品,然后输入修改后的产品信息,最后点击修改按钮。在后台,可以使用servlet接收表单数据,然后使用Mybatis框架更新数据库中对应的产品信息。
```java
public class UpdateProductServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
private ProductDao productDao = new ProductDaoImpl();
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int id = Integer.parseInt(request.getParameter("id"));
String name = request.getParameter("name");
double price = Double.parseDouble(request.getParameter("price"));
String description = request.getParameter("description");
Product product = new Product();
product.setId(id);
product.setName(name);
product.setPrice(price);
product.setDescription(description);
boolean result = productDao.updateProduct(product);
if(result) {
response.sendRedirect("productList.jsp");
} else {
response.sendRedirect("updateProduct.jsp?id=" + id);
}
}
}
```
3.5 查询产品信息
在查询产品信息页面中,用户可以选择需要查询的产品,然后点击查询按钮。在后台,可以使用Mybatis框架查询数据库中对应的产品信息,并将结果返回到前台页面显示。
```java
public class QueryProductServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
private ProductDao productDao = new ProductDaoImpl();
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String name = request.getParameter("name");
List<Product> productList = productDao.getProductListByName(name);
request.setAttribute("productList", productList);
request.getRequestDispatcher("productList.jsp").forward(request, response);
}
}
```
以上是一个简单的示例,希望对您有所帮助。
阅读全文
相关推荐
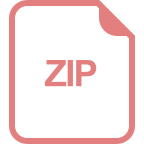
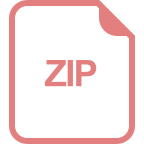
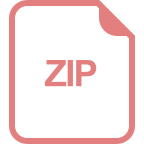















