os.path.join使用方法
时间: 2024-08-01 09:01:47 浏览: 63
`os.path.join()`是Python标准库中的os模块提供的一种函数,用于合并路径名。它能够处理Windows、Linux和Unix等不同操作系统的路径分隔符,确保生成的是平台无关的统一路径字符串。这个方法的基本用法如下:
```python
import os
# 给定两个或更多的路径组件
path1 = "parent"
path2 = "subdir"
full_path = os.path.join(path1, path2)
print(full_path) # 输出: parent\subdir (在Windows系统)
print(full_path) # 输出: parent/subdir (在大多数Unix-like系统)
```
当你需要拼接目录和文件名,或者在不确定当前工作目录的情况下构造完整路径时,可以使用`os.path.join()`. 它会自动处理必要的路径分隔符,并且支持空字符串作为前导路径,以适应相对路径的情况。
相关问题
将下面代码简洁化:def split_dataset(img_path, target_folder_path, output_path): filename = [] total_imgs = os.listdir(img_path) #for root, dirs, files in os.walk(img_path): for img in total_imgs: filename.append(img) np.random.shuffle(filename) train = filename[:int(len(filename) * 0.9)] test = filename[int(len(filename) * 0.9):] out_images = os.path.join(output_path, 'imgs') if not os.path.exists(out_images): os.makedirs(out_images) out_images_train = os.path.join(out_images, 'training') if not os.path.exists(out_images_train): os.makedirs(out_images_train) out_images_test = os.path.join(out_images, 'test') if not os.path.exists(out_images_test): os.makedirs(out_images_test) out_annotations = os.path.join(output_path, 'annotations') if not os.path.exists(out_annotations): os.makedirs(out_annotations) out_annotations_train = os.path.join(out_annotations, 'training') if not os.path.exists(out_annotations_train): os.makedirs(out_annotations_train) out_annotations_test = os.path.join(out_annotations, 'test') if not os.path.exists(out_annotations_test): os.makedirs(out_annotations_test) for i in train: print(os.path.join(img_path, i)) print(os.path.join(out_images_train, i)) shutil.copyfile(os.path.join(img_path, i), os.path.join(out_images_train, i)) annotations_name = "gt_" + i[:-3] + 'txt' shutil.copyfile(os.path.join(target_folder_path, annotations_name), os.path.join(out_annotations_train, annotations_name)) for i in test: shutil.copyfile(os.path.join(img_path, i), os.path.join(out_images_test, i)) annotations_name = "gt_" + i[:-3] + 'txt' shutil.copyfile(os.path.join(target_folder_path, annotations_name), os.path.join(out_annotations_test, annotations_name))
def split_dataset(img_path, target_folder_path, output_path):
filename = os.listdir(img_path)
np.random.shuffle(filename)
train = filename[:int(len(filename) * 0.9)]
test = filename[int(len(filename) * 0.9):]
out_images = os.path.join(output_path, 'imgs')
os.makedirs(out_images, exist_ok=True)
out_images_train = os.path.join(out_images, 'training')
os.makedirs(out_images_train, exist_ok=True)
out_images_test = os.path.join(out_images, 'test')
os.makedirs(out_images_test, exist_ok=True)
out_annotations = os.path.join(output_path, 'annotations')
os.makedirs(out_annotations, exist_ok=True)
out_annotations_train = os.path.join(out_annotations, 'training')
os.makedirs(out_annotations_train, exist_ok=True)
out_annotations_test = os.path.join(out_annotations, 'test')
os.makedirs(out_annotations_test, exist_ok=True)
for i in train:
img_src = os.path.join(img_path, i)
img_dst = os.path.join(out_images_train, i)
shutil.copyfile(img_src, img_dst)
annotations_name = "gt_" + i[:-3] + 'txt'
annotations_src = os.path.join(target_folder_path, annotations_name)
annotations_dst = os.path.join(out_annotations_train, annotations_name)
shutil.copyfile(annotations_src, annotations_dst)
for i in test:
img_src = os.path.join(img_path, i)
img_dst = os.path.join(out_images_test, i)
shutil.copyfile(img_src, img_dst)
annotations_name = "gt_" + i[:-3] + 'txt'
annotations_src = os.path.join(target_folder_path, annotations_name)
annotations_dst = os.path.join(out_annotations_test, annotations_name)
shutil.copyfile(annotations_src, annotations_dst)
修改下列代码的错误pns_folder = os.path.join(src_folder, "PNS Files") os.makedirs(pns_folder) bg_folder = os.path.join(src_folder, "BG Files") os.makedirs(bg_folder) # 初始化文件夹,用于计数移动了多少文件夹 PNS_files_count = 0 BG_files_count = 0 # 遍历目标文件夹中的文件夹和文件 for root, dirs, files in os.walk(src_folder): for dir in dirs: if PNS_keyword in dir: os.makedirs(os.path.join(pns_folder, dir)) elif BG_keyword in dir: os.makedirs(os.path.join(bg_folder, dir)) for filename in files: file_path = os.path.join(root, filename) if os.path.isfile(file_path): if PNS_keyword in filename: folder_path = os.path.join(pns_folder, os.path.basename(os.path.dirname(file_path))) os.makedirs(folder_path, exist_ok=True) os.rename(file_path, os.path.join(folder_path, filename)) PNS_files_count += 1 elif BG_keyword in filename: folder_path = os.path.join(bg_folder, os.path.basename(os.path.dirname(file_path))) os.makedirs(folder_path, exist_ok=True) os.rename(file_path, os.path.join(folder_path, filename)) BG_files_count += 1 # 计算移动了多少文件夹 a1 = PNS_files_count / 2 b1 = BG_files_count / 2
这段代码的错误在于缺少了PNS_keyword和BG_keyword的定义,需要在代码中进行定义。同时,还需要判断目标文件夹中是否已经存在PNS Files和BG Files文件夹,如果已经存在,则不再创建。
修改后的代码如下:
```
import os
PNS_keyword = "PNS" # 定义PNS关键字
BG_keyword = "BG" # 定义BG关键字
pns_folder = os.path.join(src_folder, "PNS Files")
if not os.path.exists(pns_folder):
os.makedirs(pns_folder)
bg_folder = os.path.join(src_folder, "BG Files")
if not os.path.exists(bg_folder):
os.makedirs(bg_folder)
# 初始化文件夹,用于计数移动了多少文件夹
PNS_files_count = 0
BG_files_count = 0
# 遍历目标文件夹中的文件夹和文件
for root, dirs, files in os.walk(src_folder):
for dir in dirs:
if PNS_keyword in dir:
pns_sub_folder = os.path.join(pns_folder, dir)
if not os.path.exists(pns_sub_folder):
os.makedirs(pns_sub_folder)
elif BG_keyword in dir:
bg_sub_folder = os.path.join(bg_folder, dir)
if not os.path.exists(bg_sub_folder):
os.makedirs(bg_sub_folder)
for filename in files:
file_path = os.path.join(root, filename)
if os.path.isfile(file_path):
if PNS_keyword in filename:
folder_path = os.path.join(pns_folder, os.path.basename(os.path.dirname(file_path)))
os.makedirs(folder_path, exist_ok=True)
os.rename(file_path, os.path.join(folder_path, filename))
PNS_files_count += 1
elif BG_keyword in filename:
folder_path = os.path.join(bg_folder, os.path.basename(os.path.dirname(file_path)))
os.makedirs(folder_path, exist_ok=True)
os.rename(file_path, os.path.join(folder_path, filename))
BG_files_count += 1
# 计算移动了多少文件夹
a1 = PNS_files_count / 2
b1 = BG_files_count / 2
```
这个修改后的代码在前面加入了PNS_keyword和BG_keyword的定义,并且在创建PNS Files和BG Files文件夹之前,判断它们是否已经存在,如果已经存在,则不再创建。
阅读全文
相关推荐
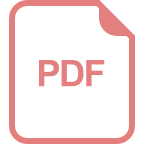
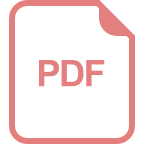
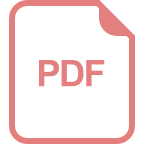













