安卓如何post一个json并接收返回的json信息
时间: 2024-05-05 17:15:13 浏览: 100
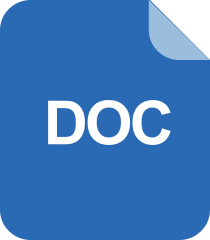
android中post获取json

可以使用Android中的HttpClient或者Volley库来实现POST请求并接收返回的JSON信息。
1. 使用HttpClient
HttpClient已经过时,但是仍然可以使用。以下是一个示例代码:
```
try {
HttpClient httpClient = new DefaultHttpClient();
HttpPost httpPost = new HttpPost(url);
// 设置请求头
httpPost.setHeader("Content-Type", "application/json");
httpPost.setHeader("Accept", "application/json");
// 构造JSON数据
JSONObject jsonObject = new JSONObject();
jsonObject.put("key1", "value1");
jsonObject.put("key2", "value2");
StringEntity stringEntity = new StringEntity(jsonObject.toString());
// 设置请求体
httpPost.setEntity(stringEntity);
// 发送请求
HttpResponse httpResponse = httpClient.execute(httpPost);
// 获取返回的JSON信息
BufferedReader reader = new BufferedReader(new InputStreamReader(httpResponse.getEntity().getContent()));
StringBuffer stringBuffer = new StringBuffer();
String line = "";
while ((line = reader.readLine()) != null) {
stringBuffer.append(line);
}
// 处理返回的JSON信息
String response = stringBuffer.toString();
JSONObject responseJson = new JSONObject(response);
} catch (Exception e) {
e.printStackTrace();
}
```
2. 使用Volley
Volley是一个网络请求库,可以更轻松地发送网络请求。以下是一个示例代码:
```
try {
RequestQueue requestQueue = Volley.newRequestQueue(context);
String url = "http://example.com/api";
JSONObject jsonBody = new JSONObject();
jsonBody.put("key1", "value1");
jsonBody.put("key2", "value2");
final String requestBody = jsonBody.toString();
JsonObjectRequest jsonObjectRequest = new JsonObjectRequest(Request.Method.POST, url, null, new Response.Listener<JSONObject>() {
@Override
public void onResponse(JSONObject response) {
// 处理返回的JSON信息
}
}, new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
error.printStackTrace();
}
}) {
@Override
public String getBodyContentType() {
return "application/json; charset=utf-8";
}
@Override
public byte[] getBody() {
try {
return requestBody == null ? null : requestBody.getBytes("utf-8");
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
return null;
}
}
};
requestQueue.add(jsonObjectRequest);
} catch (JSONException e) {
e.printStackTrace();
}
```
以上代码仅供参考,具体实现可能需要根据具体情况进行调整。
阅读全文
相关推荐

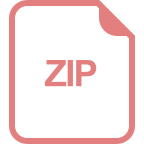
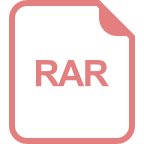
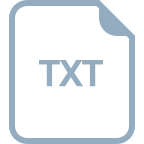
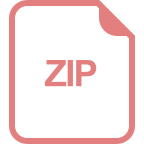
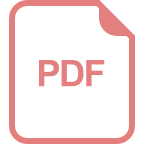
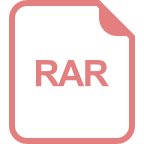
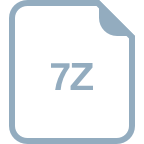
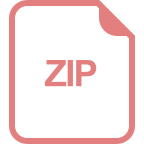
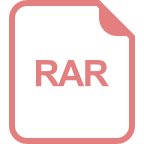
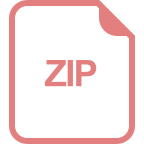
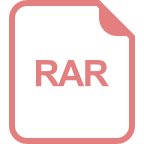
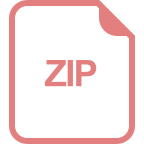
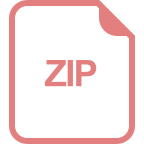
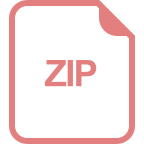