1、将编号为0和1的两个栈存放于一个数组空间V[m]中,栈底分别处于数组的两端。当第0号栈的栈顶指针top[0]等于-1时该栈为空;当第1号栈的栈顶指针top[1]等于m时,该栈为空。两个栈均从两端向中间增长。试编写双栈初始化,判断栈空、栈满、进栈和出栈等算法的函数。C++
时间: 2024-12-06 15:22:02 浏览: 17
为了实现这种双栈结构,我们可以创建两个指针 top0 和 top1,分别表示每个栈的栈顶。由于栈底位于数组的两端,我们还需要一个额外的信息来确定当前正在使用的栈。我们可以设置一个整数 currentStack,初始值为0,表示使用的是编号为0的栈。
以下是初始化以及基本操作的C++函数:
```cpp
#include <vector>
// 初始化双栈
void initDoubleStack(std::vector<int>& V, int m) {
V.resize(m * 2); // 创建大小为m*2的数组
for (int i = 0; i < m; ++i) { // 初始化两个栈的栈底
V[i] = -1;
V[m + i] = m;
}
}
// 判断栈是否为空
bool isEmpty(int& top0, int& top1, int currentStack) {
return currentStack == 0 && top0 == -1 || currentStack == 1 && top1 == m;
}
// 判断栈是否已满
bool isFull(int& top0, int& top1, int currentStack) {
return currentStack == 0 && top0 == m / 2 || currentStack == 1 && top1 == m * 1.5;
}
// 进栈操作
void push(int& top0, int& top1, int value, int& currentStack) {
if (!isFull(top0, top1, currentStack)) {
if (currentStack == 0) {
top0++;
V[top0] = value;
} else { // currentStack == 1
top1++;
V[top1] = value;
}
} else {
// 栈满提示错误
std::cout << "Error: Stack overflow!" << std::endl;
}
currentStack = 1 - currentStack; // 交替使用两个栈
}
// 出栈操作
int pop(int& top0, int& top1, int currentStack) {
if (!isEmpty(top0, top1, currentStack)) {
int value;
if (currentStack == 0) {
value = V[top0];
top0--;
} else { // currentStack == 1
value = V[top1];
top1--;
}
return value;
} else {
// 栈空提示错误
std::cout << "Error: Stack underflow!" << std::endl;
return -1; // 返回特殊值表示失败
}
}
```
在这个实现中,`initDoubleStack()` 函数初始化了存储空间,`isEmpty()`, `isFull()`, `push()`, 和 `pop()` 分别处理栈空、栈满和进出栈的操作。注意,在进行出栈时,我们需要检查栈是否为空,因为如果试图弹出空栈,程序需要返回错误信息。
阅读全文
相关推荐
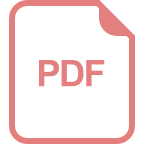
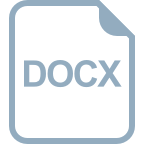
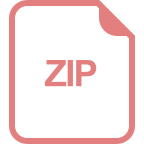
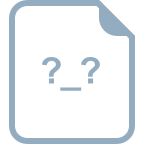
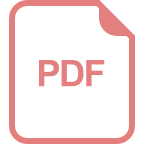
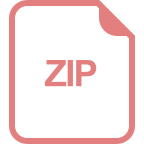
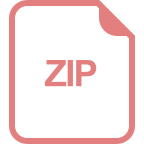
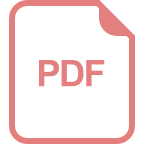
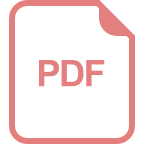
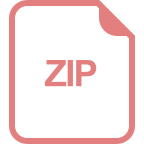
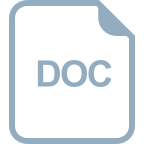
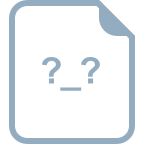
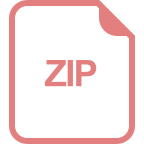
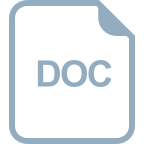
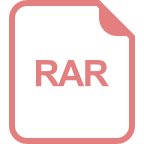
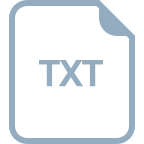
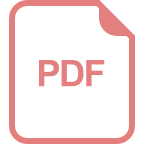
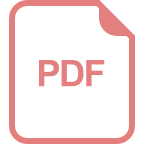