学生成绩管理系统菜单
时间: 2023-12-15 17:06:17 浏览: 30
以下是一个用结构体数组作为函数参数的学生成绩管理系统菜单驱动的例子:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define COURSE_NUM 3 // 课程数
#define STUDENT_NUM 3 // 学生数
// 学生结构体
typedef struct {
char id[20]; // 学号
char name[20]; // 姓名
float score[COURSE_NUM]; // 成绩
float totalScore; // 总分
float avgScore; // 平均分
} Student;
// 录入学生信息
void input(Student *students, int n) {
for (int i = 0; i < n; i++) {
printf("请输入第%d个学生的学号:", i + 1);
scanf("%s", students[i].id);
printf("请输入第%d个学生的姓名:", i + 1);
scanf("%s", students[i].name);
for (int j = 0; j < COURSE_NUM; j++) {
printf("请输入第%d个学生的第%d门课程成绩:", i + 1, j + 1);
scanf("%f", &students[i].score[j]);
}
}
}
// 计算每门课程的总分和平均分
void calcCourseScore(Student *students, int n) {
float totalScore[COURSE_NUM] = {0}; // 每门课程的总分
float avgScore[COURSE_NUM] = {0}; // 每门课程的平均分
for (int i = 0; i < n; i++) {
for (int j = 0; j < COURSE_NUM; j++) {
totalScore[j] += students[i].score[j];
}
}
for (int i = 0; i < COURSE_NUM; i++) {
avgScore[i] = totalScore[i] / n;
printf("第%d门课程的总分为%.2f,平均分为%.2f\n", i + 1, totalScore[i], avgScore[i]);
}
}
// 计算每个学生的总分和平均分
void calcStudentScore(Student *students, int n) {
for (int i = 0; i < n; i++) {
students[i].totalScore = 0;
for (int j = 0; j < COURSE_NUM; j++) {
students[i].totalScore += students[i].score[j];
}
students[i].avgScore = students[i].totalScore / COURSE_NUM;
printf("%s的总分为%.2f,平均分为%.2f\n", students[i].name, students[i].totalScore, students[i].avgScore);
}
}
// 按每个学生的总分由高到低排序
void sort(Student *students, int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (students[j].totalScore < students[j + 1].totalScore) {
Student temp = students[j];
students[j] = students[j + 1];
students[j + 1] = temp;
}
}
}
for (int i = 0; i < n; i++) {
printf("%s的总分为%.2f,平均分为%.2f\n", students[i].name, students[i].totalScore, students[i].avgScore);
}
}
// 主菜单
void mainMenu(Student *students, int n) {
int choice;
while (1) {
printf("请选择操作:\n");
printf("1. 录入学生信息\n");
printf("2. 计算每门课程的总分和平均分\n");
printf("3. 计算每个学生的总分和平均分\n");
printf("4. 按每个学生的总分由高到低排序\n");
printf("5. 退出\n");
scanf("%d", &choice);
switch (choice) {
case 1:
input(students, n);
break;
case 2:
calcCourseScore(students, n);
break;
case 3:
calcStudentScore(students, n);
break;
case 4:
sort(students, n);
break;
case 5:
exit(0);
default:
printf("输入有误,请重新输入!\n");
break;
}
}
}
int main() {
Student students[STUDENT_NUM];
mainMenu(students, STUDENT_NUM);
return 0;
}
```
阅读全文
相关推荐
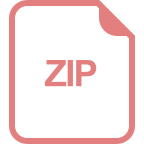
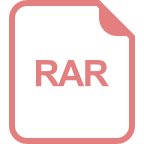
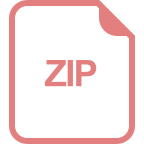
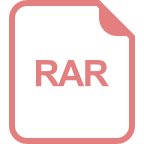
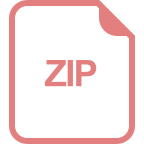
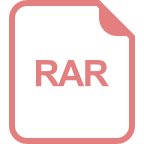
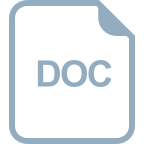
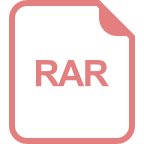
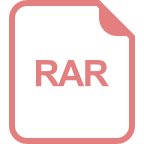
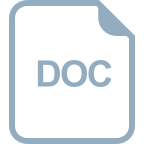
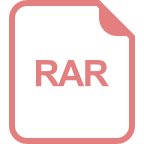
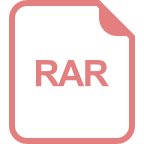
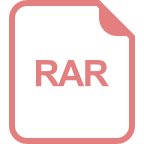
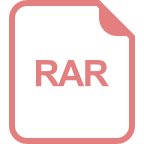