pyqt5 圆盘按钮 四个方向
时间: 2024-12-25 11:08:00 浏览: 2
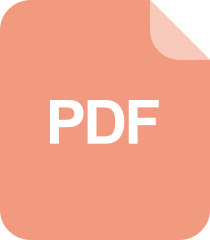
pyqt5使用按钮进行界面的跳转方法
PyQt5是一个基于Python的GUI应用程序开发框架,它封装了Qt库,使得在Python中创建用户界面变得简单。圆盘按钮通常是指带有圆形边缘并可以向四个基本方向(上、下、左、右)切换的控件,这在界面上常用于指示方向或提供选择。
在PyQt5中,你可以通过`QToolButton`或者`QPushButton`结合`setStyleSheet`方法以及CSS样式来模拟这种效果。例如,你可以创建一个普通的工具按钮,然后设置其背景图像为一个带箭头的圆盘,通过改变其`border-radius`属性使其呈现圆形,并调整鼠标悬停和按下状态下的图像变化,来模拟按压的方向感。
下面是一个简单的例子:
```python
from PyQt5.QtWidgets import QApplication, QToolButton, QVBoxLayout
from PyQt5.QtGui import QPainter, QColor, QPixmap
class DiskButton(QToolButton):
def paintEvent(self, event):
painter = QPainter(self)
painter.setRenderHint(QPainter.Antialiasing) # 使用抗锯齿渲染提高美观度
color = QColor("#ffffff") if self.isChecked() else QColor("#000000")
radius = min(self.width(), self.height()) / 2 - 2 # 计算半径
painter.setPen(color)
painter.drawRoundedRect(event.rect(), radius, radius)
# 创建圆盘按钮实例
button = DiskButton()
button.setText("上")
button.setStyleSheet("""
background-image: url(path_to_your_arrow_image);
border-radius: %dpx;
""" % (radius * 2)) # 设置边框半径
# 方向设置
directions = ["上", "下", "左", "右"]
for i in range(4):
direction_button = DiskButton()
direction_button.setText(directions[i])
# 更改图片路径和文本内容
direction_button.setStyleSheet(
f"""
background-image: url(path_to_your_{directions[i]}_arrow_image);
"""
)
layout = QVBoxLayout()
layout.addWidget(direction_button)
# 根据需要添加到窗口布局中
app = QApplication([])
main_window = QWidget()
main_window.setLayout(layout)
main_window.show()
app.exec_()
```
别忘了替换`path_to_your_*_arrow_image`为实际包含相应箭头图标文件的URL或本地路径。
阅读全文
相关推荐
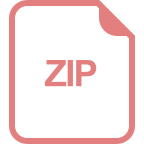
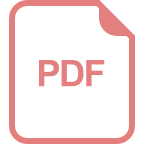
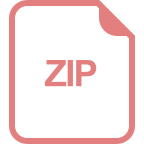
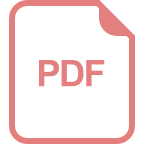
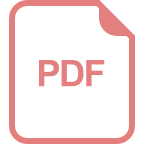
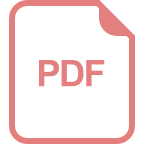
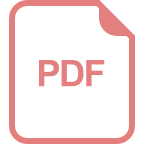
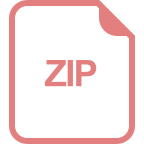
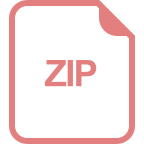
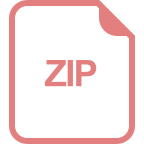