android 一个圆点沿着imageview外框转圈
时间: 2024-09-27 19:04:38 浏览: 6
在Android中,为了实现一个圆点沿着ImageView的边界循环移动的效果,我们可以利用`Canvas`绘制动态路径,并结合`Thread`或`Handler`来定期更新这个位置。这里有一个简单的示例,它会在一个圆形区域内绘制一个小圆点,使其沿着ImageView的边框移动:
首先,你需要创建一个自定义的View,然后在`onDraw()`方法中处理绘图:
```java
public class RotatingDotView extends View {
private Paint paint;
private float dotRadius;
private float dotX, dotY;
private Path path;
private boolean isAnimating;
public RotatingDotView(Context context) {
super(context);
init();
}
public RotatingDotView(Context context, @Nullable AttributeSet attrs) {
super(context, attrs);
init();
}
public RotatingDotView(Context context, @Nullable AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
init();
}
private void init() {
paint = new Paint(Paint.ANTI_ALIAS_FLAG);
paint.setColor(Color.RED); // 设置圆点颜色
dotRadius = 5; // 圆点半径
path = new Path(); // 初始化路径
isAnimating = true;
startAnimation();
}
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
if (isAnimating) {
// 计算并绘制新的圆点位置
float leftBound = getLeft() + getWidth() - dotRadius;
float topBound = getTop() + getHeight() - dotRadius;
path.reset();
path.arcTo(new RectF(getLeft(), getTop(), leftBound, topBound), 0, 360);
canvas.drawPath(path, paint);
dotX += 5; // 每次移动步长
dotX %= leftBound - dotRadius; // 确保不会超出边界
dotY = dotX; // 圆点始终在同一水平线上
invalidate(); // 强制下一次重绘
}
}
public void stopAnimation() {
isAnimating = false;
}
// 可选的方法,用于启动动画(通常放在布局文件的onclick监听器)
public void startAnimation() {
new Thread(() -> {
while (isAnimating) {
try {
Thread.sleep(50); // 调整间隔以改变速度
} catch (InterruptedException e) {
e.printStackTrace();
}
postInvalidate();
}
}).start();
}
}
```
然后,在XML布局中添加此自定义View,并为其设置点击事件以便启动或停止动画:
```xml
<Button
android:id="@+id/start_stop_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Start Animation"
android:onClick="toggleAnimation"/>
<com.example.RotatingDotView
android:id="@+id/dot_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true" />
```
相关推荐
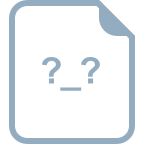
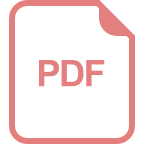
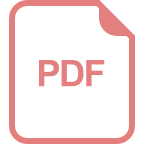














