mybatis yaml文件配置typeAliasesPackage怎么跳过指定的包名
时间: 2024-04-22 10:24:43 浏览: 155
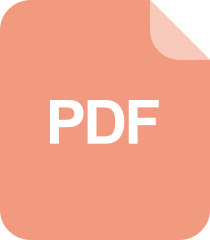
MyBatis 如何写配置文件和简单使用
在 MyBatis 的 YAML 配置文件中,要跳过指定的包名,可以使用 `!` 符号来排除特定的包。具体步骤如下:
1. 打开 MyBatis 的 YAML 配置文件。
2. 定位到 `typeAliasesPackage` 属性的配置行。
3. 在包名字符串之前添加 `!` 符号,表示要排除该包名。
4. 保存配置文件。
以下是一个示例,演示如何跳过指定的包名:
```yaml
mybatis:
configuration:
typeAliasesPackage: "!com.example.exclude.package.*,com.example.include.package.*"
```
在上述示例中,`com.example.exclude.package.*` 是要跳过的包名,而 `com.example.include.package.*` 是要包含的包名。通过使用 `!` 符号,可以排除指定的包名。
请注意,上述示例仅适用于 MyBatis 的 YAML 配置文件。如果你使用的是 XML 配置文件,则需要稍作调整,将 `typeAliasesPackage` 属性的值设置为 `!com.example.exclude.package.*,com.example.include.package.*`。
阅读全文
相关推荐
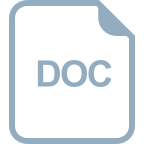
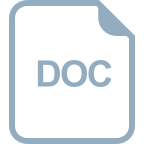
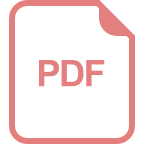
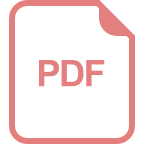
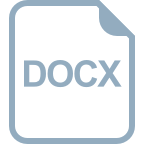
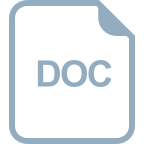
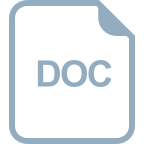
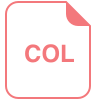
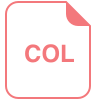








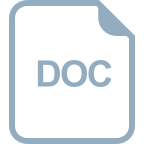