qiankun中使用vuex进行父子通信
时间: 2024-01-08 12:04:57 浏览: 142
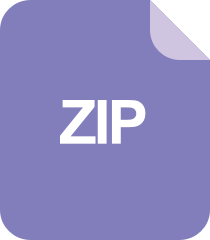
华为乾坤安全云服务 产品文档(chm)

在qiankun中,主应用和子应用之间可以通过vuex进行状态管理和通信。以下是使用vuex在主应用和子应用之间进行通信的步骤:
1. 在主应用中,创建一个共享状态的vuex store,并将其传递给子应用。
```javascript
// 主应用中的store.js
import Vue from 'vue';
import Vuex from 'vuex';
Vue.use(Vuex);
const store = new Vuex.Store({
state: {
message: '',
},
mutations: {
setMessage(state, message) {
state.message = message;
},
},
});
export default store;
```
```javascript
// 主应用中的main.js
import Vue from 'vue';
import App from './App.vue';
import store from './store';
new Vue({
store,
render: h => h(App),
}).$mount('#app');
// 注册微应用
registerMicroApps([
{
name: 'sub-app',
entry: '//localhost:8080',
container: '#subapp-container',
activeRule: '/sub-app',
props: {
globalStore: store,
},
},
]);
```
2. 在子应用中,接收主应用传递过来的store,并在子应用中使用store中的状态和mutations。
```javascript
// 子应用中的main.js
import Vue from 'vue';
import App from './App.vue';
let instance = null;
function render(props = {}) {
const { globalStore } = props;
new Vue({
render: h => h(App, { props: { globalStore } }),
}).$mount('#app');
}
if (!window.__POWERED_BY_QIANKUN__) {
render();
}
export async function bootstrap() {}
export async function mount(props) {
instance = render(props);
}
export async function unmount() {
instance.$destroy();
instance = null;
}
```
```javascript
// 子应用中的App.vue
<template>
<div id="app">
<h1>Sub App</h1>
<p>{{ message }}</p>
<button @click="updateMessage">Update Message</button>
</div>
</template>
<script>
export default {
props: {
globalStore: Object,
},
computed: {
message() {
return this.globalStore.state.message;
},
},
methods: {
updateMessage() {
this.globalStore.commit('setMessage', 'Hello from sub app!');
},
},
};
</script>
```
在子应用中,通过props接收主应用传递过来的store,并在computed中使用store中的状态,在methods中使用store中的mutations。这样,主应用和子应用就可以共享vuex store中的状态,并通过mutations进行通信了。
阅读全文
相关推荐
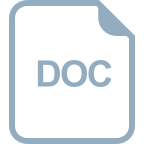
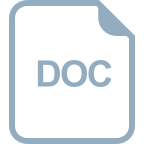
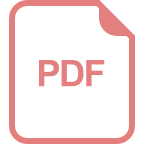
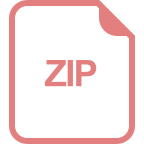
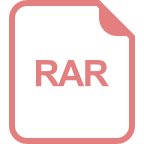
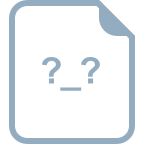
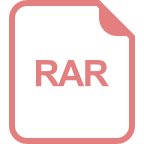
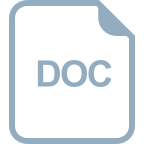
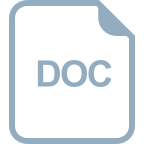
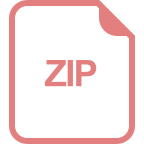
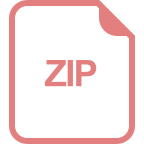
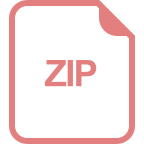