electron 利用child_process如何获取使用第三方程序的时间
时间: 2024-05-16 17:16:57 浏览: 8
你可以使用Node.js中的child_process模块来启动第三方程序并监视其运行时间。以下是一个简单的示例代码:
```javascript
const { spawn } = require('child_process');
// 启动第三方程序
const childProcess = spawn('your-program.exe', ['arg1', 'arg2']);
// 记录启动时间
const startTime = new Date();
// 监听程序退出事件
childProcess.on('exit', (code) => {
const endTime = new Date();
const elapsedTime = endTime - startTime;
console.log(`程序运行时间:${elapsedTime}毫秒`);
});
```
在上面的示例中,我们使用spawn函数启动了一个名为"your-program.exe"的第三方程序,并传递了一些命令行参数。然后,我们记录了程序的启动时间,并在程序退出时计算了运行时间。最后,我们将运行时间输出到控制台。你可以根据自己的需要对代码进行修改以满足特定的需求。
相关问题
electron 利用child_process命令行exec如何获取打开的应用关闭事件
使用child_process模块的exec函数启动一个应用程序后,无法直接获取该应用程序关闭的事件。这是因为exec函数只是启动了一个子进程,并不会监控该应用程序的状态。
如果你想要获取应用程序关闭的事件,可以考虑使用Node.js的`process`模块的`on`函数来监听进程关闭事件。具体步骤如下:
1. 使用child_process模块的exec函数启动应用程序,例如:
```javascript
const { exec } = require("child_process");
exec("notepad.exe", (error, stdout, stderr) => {
if (error) {
console.error(`exec error: ${error}`);
return;
}
console.log(`stdout: ${stdout}`);
console.error(`stderr: ${stderr}`);
});
```
2. 在子进程启动后,使用`process`模块的`on`函数监听`exit`事件,例如:
```javascript
const { exec } = require("child_process");
const child = exec("notepad.exe", (error, stdout, stderr) => {
if (error) {
console.error(`exec error: ${error}`);
return;
}
console.log(`stdout: ${stdout}`);
console.error(`stderr: ${stderr}`);
});
child.on("exit", (code) => {
console.log(`Child process exited with code ${code}`);
});
```
这样,当应用程序被关闭时,子进程也会被关闭,从而触发`exit`事件,你就可以在该事件中处理应用程序关闭的逻辑了。
electron 利用child_process spawn 的一些参数
Electron 的 child_process 模块提供了 spawn 方法,它用于启动一个新进程。spawn 方法接受多个参数,其中一些是可选的。下面是一些常用的参数:
1. command:要运行的命令或脚本文件的路径。
2. args:传递给命令或脚本的参数数组,每个元素都是一个字符串。
3. options:一个对象,其属性是可选的,用于控制子进程的行为。常用的属性包括:
- cwd:设置子进程的当前工作目录。
- env:设置子进程的环境变量。
- stdio:控制子进程的标准输入、输出和错误输出。可以设置为 'pipe'(创建一个管道)、'ignore'(忽略)、'inherit'(继承)或一个数组,数组中每个元素对应一个文件描述符。
- detached:设置为 true 时,子进程会变成一个独立的进程组,不受父进程的控制。
- uid、gid:设置子进程的用户 ID 和组 ID。
下面是一个示例代码:
```javascript
const { spawn } = require('child_process');
const ls = spawn('ls', ['-lh', '/usr']);
ls.stdout.on('data', (data) => {
console.log(`stdout: ${data}`);
});
ls.stderr.on('data', (data) => {
console.error(`stderr: ${data}`);
});
ls.on('close', (code) => {
console.log(`child process exited with code ${code}`);
});
```
这个示例代码启动了一个 ls 命令,列出了 /usr 目录下的文件。spawn 方法返回一个 ChildProcess 对象,可以通过它来监听子进程的事件,如 stdout、stderr 和 close。
相关推荐
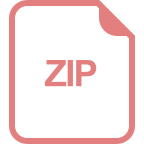












