多线程代码一般用在什么场景,如何保证线程变量安全?写一个多线程的例子
时间: 2024-09-23 18:11:19 浏览: 45
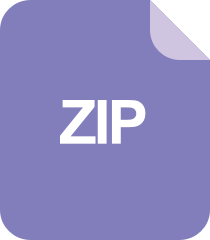
商业编程-源码-一个用多线程实现文件查找的例子.zip
多线程代码通常用在需要并发执行任务的场景,例如网络请求、用户界面更新、数据处理等,它可以提高程序的响应速度和资源利用率。然而,当多个线程访问共享的数据时,如果没有适当的同步措施,可能会导致数据竞争(race condition),破坏程序的正确性和一致性。
保证线程变量安全通常采用以下几种技术:
1. **锁(Locks)**: 使用互斥锁(如Java的`synchronized`关键字或Python的`threading.Lock`)可以防止多个线程同时修改同一块数据。
2. **原子操作(Atomic Operations)**: 一些语言提供原子操作,它们能保证在单个操作下不会被打断,比如Java的`AtomicInteger`。
3. ** volatile 关键字/属性(Volatile)**: 保证了对共享变量的读取不会缓存,使得多线程环境下的可见性。
4. **线程局部存储(Thread Local Storage, TLS)**: 每个线程有自己的副本,避免了数据竞争。
下面是一个简单的Java多线程示例,使用了`synchronized`关键字保证线程安全:
```java
class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public synchronized int getCount() {
return count;
}
}
public class Main {
public static void main(String[] args) throws InterruptedException {
Counter counter = new Counter();
Thread thread1 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
counter.increment();
}
});
Thread thread2 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
counter.increment();
}
});
thread1.start();
thread2.start();
// 等待两个线程结束
thread1.join();
thread2.join();
System.out.println(counter.getCount()); // 输出应该是2000,因为每个线程都独立增了一次
}
}
```
在这个例子中,`increment`和`getCount`方法都被标记为`synchronized`,这意味着在同一时刻只能有一个线程执行这两个方法,从而保证了线程变量的安全性。
阅读全文
相关推荐
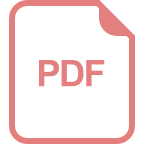
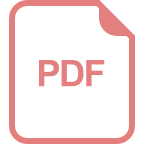
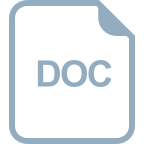
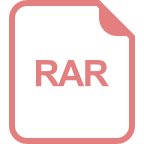
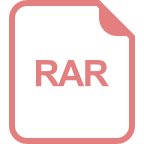
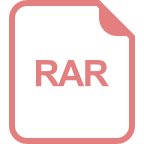
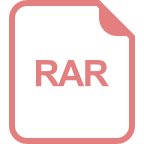
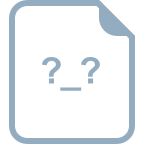
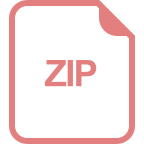
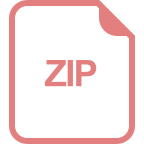
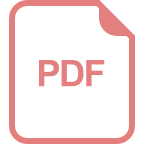
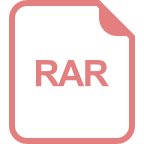
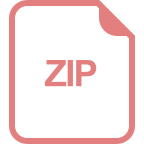
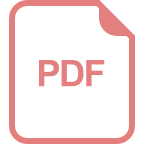
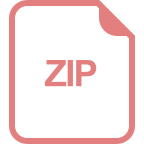
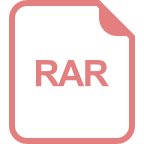
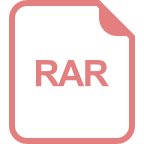