使用springboot 实现一个nginx反向代理的类
时间: 2024-06-12 11:10:50 浏览: 13
好的,以下是使用 Spring Boot 实现一个 Nginx 反向代理的类:
```java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.Bean;
import org.springframework.web.client.RestTemplate;
@SpringBootApplication
public class ReverseProxyApplication {
public static void main(String[] args) {
SpringApplication.run(ReverseProxyApplication.class, args);
}
@Bean
public RestTemplate restTemplate() {
return new RestTemplate();
}
}
```
这个类使用了 Spring Boot 框架,其中包含了一个 `RestTemplate` 的 Bean,用于发送 HTTP 请求。在实际使用中,我们可以通过注入 `RestTemplate` 来发送请求。
接下来,我们需要编写一个 Controller 类,用于接收请求并将其转发到目标服务器:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.client.RestTemplate;
@RestController
public class ReverseProxyController {
@Autowired
private RestTemplate restTemplate;
@GetMapping("/{path}")
public ResponseEntity<String> proxy(@PathVariable String path) {
String url = "http://example.com/" + path;
ResponseEntity<String> response = restTemplate.getForEntity(url, String.class);
return response;
}
}
```
这个 Controller 类中包含了一个 `RestTemplate` 的实例,用于发送 HTTP 请求。在 `proxy` 方法中,我们将请求路径拼接到目标服务器的 URL 上,并使用 `RestTemplate` 发送 GET 请求。最后,我们将目标服务器的响应返回给客户端。
以上就是使用 Spring Boot 实现一个 Nginx 反向代理的类的全部内容。
相关推荐
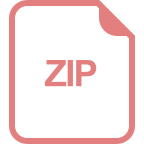














