如何用Java语言将多个级别的hashmap结果分类为多个文件夹,并从文件夹中返回原有值,里面包括info
时间: 2024-02-12 12:02:26 浏览: 97
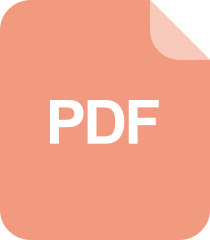
java实现从方法返回多个值功能示例
可以通过以下步骤实现:
1. 遍历多层嵌套的 HashMap,将每个 HashMap 中的信息提取出来,并生成对应的文件夹路径。
2. 将提取出来的信息写入到对应的文件夹中,可以使用 Java 的文件操作类(如 File、BufferedWriter 等)实现。
3. 在写入信息时,可以将原有的信息以 JSON 格式存储,方便后续读取。
4. 当需要获取原有值时,通过读取对应的文件夹中的 JSON 文件,将其转化为 Java 对象即可。
下面是代码示例:
```java
import java.io.*;
import java.util.HashMap;
import java.util.Map;
public class HashmapToFile {
public static void main(String[] args) throws IOException {
Map<String, Object> map = new HashMap<>();
map.put("name", "张三");
map.put("age", 20);
Map<String, Object> subMap = new HashMap<>();
subMap.put("name", "李四");
subMap.put("age", 25);
Map<String, Object> data = new HashMap<>();
data.put("info", map);
data.put("subInfo", subMap);
String basePath = "/path/to/base/folder";
writeDataToFolder(basePath, data);
// 读取数据
Map<String, Object> result = readDataFromFolder(basePath);
System.out.println(result);
}
/**
* 将数据写入到对应的文件夹中
*
* @param basePath 文件夹基础路径
* @param data 待写入的数据
* @throws IOException IO异常
*/
public static void writeDataToFolder(String basePath, Map<String, Object> data) throws IOException {
for (Map.Entry<String, Object> entry : data.entrySet()) {
String folderPath = basePath + "/" + entry.getKey();
File folder = new File(folderPath);
if (!folder.exists()) {
folder.mkdirs();
}
// 将数据转换为 JSON 字符串并写入文件
String json = JsonUtil.toJson(entry.getValue());
String filePath = folderPath + "/data.json";
BufferedWriter writer = new BufferedWriter(new FileWriter(filePath));
writer.write(json);
writer.close();
}
}
/**
* 从文件夹中读取数据
*
* @param basePath 文件夹基础路径
* @return 读取到的数据
* @throws IOException IO异常
*/
public static Map<String, Object> readDataFromFolder(String basePath) throws IOException {
Map<String, Object> result = new HashMap<>();
File folder = new File(basePath);
if (!folder.exists()) {
return result;
}
File[] subFolders = folder.listFiles();
for (File subFolder : subFolders) {
if (!subFolder.isDirectory()) {
continue;
}
String key = subFolder.getName();
String filePath = subFolder.getAbsolutePath() + "/data.json";
String json = readFile(filePath);
Object value = JsonUtil.fromJson(json, Object.class);
result.put(key, value);
}
return result;
}
/**
* 读取文件内容
*
* @param filePath 文件路径
* @return 文件内容
* @throws IOException IO异常
*/
public static String readFile(String filePath) throws IOException {
BufferedReader reader = new BufferedReader(new FileReader(filePath));
StringBuilder sb = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
sb.append(line);
}
reader.close();
return sb.toString();
}
}
class JsonUtil {
// 省略 toJson 和 fromJson 方法的实现
}
```
其中,JsonUtil 类用于将 Java 对象转换为 JSON 字符串或将 JSON 字符串转换为 Java 对象,可以使用第三方库(如 Jackson、Gson 等)实现。
阅读全文
相关推荐
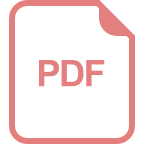
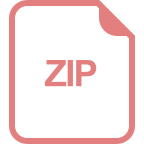
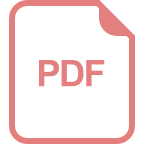
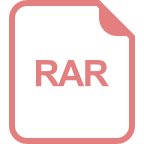
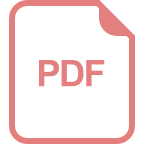
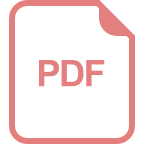
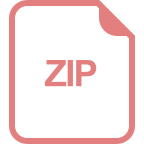
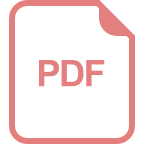
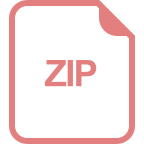
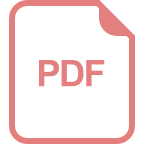
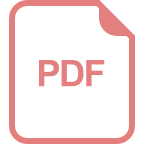
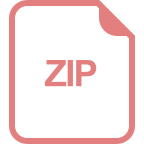
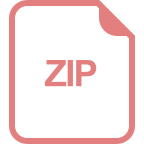
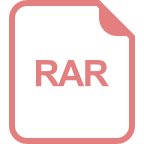
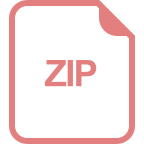