<h1>通讯录</h1> <button class="add-btn">添加联系人</button> <input type="text" id="search-input" placeholder="搜索姓名或电话"> <table> <thead> <tr> <th>姓名</th> <th>电话</th> <th>邮箱</th> <th>操作</th> </tr> </thead> <tbody id="contact-list"> <!-- 数据渲染 --> </tbody> </table> <!-- 分页按钮 --> <div id="page-buttons"></div> <!-- 弹出层,用于添加或编辑联系人 --> <div id="modal" class="modal" style="display: none;"> <div class="modal-content"> <span class="close">×</span> <form> <div class="form-group"> <label for="name">姓名</label> <input type="text" id="name" name="name" required> </div> <div class="form-group"> <label for="phone">电话</label> <input type="text" id="phone" name="phone" required> </div> <div class="form-group"> <label for="email">邮箱</label> <input type="text" id="email" name="email" required> </div> <button id="save-btn">保存</button> <button id="cancel-btn">取消</button> </form> </div> </div>帮我加入一个搜索框,通过搜索框的内容可以查找到查找的内容,让数据具有添加删除和编辑的功能,编辑的时候增加弹窗
时间: 2023-06-26 08:09:35 浏览: 50
好的,你需要在代码中添加以下功能:
1. 在搜索框上绑定一个事件监听器,当输入框中有内容输入时,筛选出匹配的联系人并进行渲染。
2. 在每个联系人的操作列中添加删除和编辑按钮,并为它们绑定事件监听器。
3. 当点击编辑按钮时,在弹出层中显示该联系人的信息,并将保存按钮改为更新按钮。
4. 当点击保存按钮时,将新的联系人信息保存到数据中并渲染页面。
5. 当点击更新按钮时,将编辑后的联系人信息更新到数据中并重新渲染页面。
下面是修改后的代码:
```html
<h1>通讯录</h1>
<button class="add-btn">添加联系人</button>
<input type="text" id="search-input" placeholder="搜索姓名或电话">
<table>
<thead>
<tr>
<th>姓名</th>
<th>电话</th>
<th>邮箱</th>
<th>操作</th>
</tr>
</thead>
<tbody id="contact-list">
<!-- 数据渲染 -->
</tbody>
</table>
<!-- 分页按钮 -->
<div id="page-buttons"></div>
<!-- 弹出层,用于添加或编辑联系人 -->
<div id="modal" class="modal" style="display: none;">
<div class="modal-content">
<span class="close">×</span>
<form>
<div class="form-group">
<label for="name">姓名</label>
<input type="text" id="name" name="name" required>
</div>
<div class="form-group">
<label for="phone">电话</label>
<input type="text" id="phone" name="phone" required>
</div>
<div class="form-group">
<label for="email">邮箱</label>
<input type="text" id="email" name="email" required>
</div>
<button id="save-btn">保存</button>
<button id="update-btn" style="display: none;">更新</button>
<button id="cancel-btn">取消</button>
</form>
</div>
</div>
<script>
// 数据
let contacts = [
{ name: "张三", phone: "13456789012", email: "zhangsan@example.com" },
{ name: "李四", phone: "15678901234", email: "lisi@example.com" },
{ name: "王五", phone: "18901234567", email: "wangwu@example.com" },
{ name: "赵六", phone: "13245678901", email: "zhaoliu@example.com" },
{ name: "钱七", phone: "17509876543", email: "qianqi@example.com" }
];
// 渲染联系人列表
function renderContacts(contacts) {
const tbody = document.getElementById("contact-list");
tbody.innerHTML = "";
contacts.forEach((contact, index) => {
const tr = document.createElement("tr");
tr.innerHTML = `
<td>${contact.name}</td>
<td>${contact.phone}</td>
<td>${contact.email}</td>
<td>
<button class="delete-btn" data-index="${index}">删除</button>
<button class="edit-btn" data-index="${index}">编辑</button>
</td>
`;
tbody.appendChild(tr);
});
}
// 添加联系人
function addContact(contact) {
contacts.push(contact);
renderContacts(contacts);
}
// 删除联系人
function deleteContact(index) {
contacts.splice(index, 1);
renderContacts(contacts);
}
// 编辑联系人
function editContact(index) {
const contact = contacts[index];
document.getElementById("name").value = contact.name;
document.getElementById("phone").value = contact.phone;
document.getElementById("email").value = contact.email;
document.getElementById("save-btn").style.display = "none";
document.getElementById("update-btn").style.display = "inline-block";
document.getElementById("modal").style.display = "block";
document.getElementById("update-btn").addEventListener("click", () => {
updateContact(index);
});
}
// 更新联系人
function updateContact(index) {
contacts[index].name = document.getElementById("name").value;
contacts[index].phone = document.getElementById("phone").value;
contacts[index].email = document.getElementById("email").value;
document.getElementById("modal").style.display = "none";
document.getElementById("update-btn").removeEventListener("click", updateContact);
renderContacts(contacts);
}
// 绑定事件监听器
document.querySelector(".add-btn").addEventListener("click", () => {
document.getElementById("name").value = "";
document.getElementById("phone").value = "";
document.getElementById("email").value = "";
document.getElementById("save-btn").style.display = "inline-block";
document.getElementById("update-btn").style.display = "none";
document.getElementById("modal").style.display = "block";
});
document.querySelector(".close").addEventListener("click", () => {
document.getElementById("modal").style.display = "none";
});
document.querySelector("#cancel-btn").addEventListener("click", () => {
document.getElementById("modal").style.display = "none";
});
document.getElementById("save-btn").addEventListener("click", (event) => {
event.preventDefault();
const contact = {
name: document.getElementById("name").value,
phone: document.getElementById("phone").value,
email: document.getElementById("email").value
};
addContact(contact);
document.getElementById("modal").style.display = "none";
});
document.getElementById("search-input").addEventListener("input", () => {
const keyword = document.getElementById("search-input").value.trim();
const filteredContacts = contacts.filter((contact) => {
return contact.name.includes(keyword) || contact.phone.includes(keyword);
});
renderContacts(filteredContacts);
});
document.getElementById("contact-list").addEventListener("click", (event) => {
const target = event.target;
if (target.classList.contains("delete-btn")) {
deleteContact(target.getAttribute("data-index"));
} else if (target.classList.contains("edit-btn")) {
editContact(target.getAttribute("data-index"));
}
});
// 初始化
renderContacts(contacts);
</script>
```
相关推荐
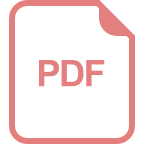
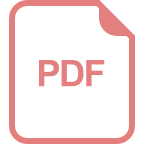
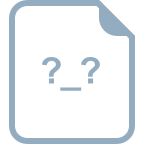




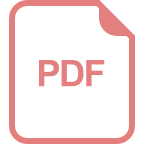
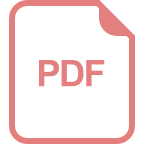
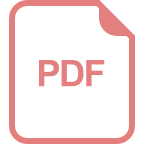
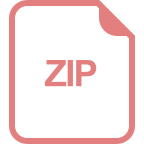
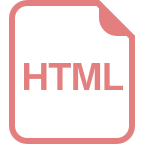
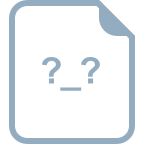
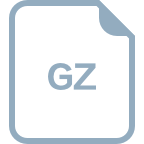