通过排除@InitBinder等注释的方式怎么实现
时间: 2024-05-05 19:21:50 浏览: 7
如果你想通过排除 `@InitBinder` 等注解的方式实现某些操作,可以使用自定义的注解来标记需要执行的方法,然后在 `WebMvcConfigurer` 中注册一个拦截器,拦截所有的请求,根据自定义注解来决定是否执行对应的方法。
步骤如下:
1. 自定义一个注解,例如 `@MyAnnotation`。
```java
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
public @interface MyAnnotation {
}
```
2. 在需要执行的方法上添加 `@MyAnnotation` 注解。
```java
@Controller
public class MyController {
@MyAnnotation
@InitBinder
public void initBinder(WebDataBinder binder) {
// ...
}
@RequestMapping("/hello")
public String hello() {
// ...
}
}
```
3. 实现一个拦截器,判断请求的方法是否有 `@MyAnnotation` 注解,如果有则执行。
```java
public class MyInterceptor implements HandlerInterceptor {
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception {
if (handler instanceof HandlerMethod) {
HandlerMethod handlerMethod = (HandlerMethod) handler;
Method method = handlerMethod.getMethod();
if (method.isAnnotationPresent(MyAnnotation.class)) {
// 执行被 @MyAnnotation 标记的方法
method.invoke(handlerMethod.getBean(), request.getParameterMap());
return false;
}
}
return true;
}
}
```
4. 在 `WebMvcConfigurer` 中注册拦截器。
```java
@Configuration
public class WebMvcConfig implements WebMvcConfigurer {
@Override
public void addInterceptors(InterceptorRegistry registry) {
registry.addInterceptor(new MyInterceptor()).addPathPatterns("/**");
}
}
```
这样就可以实现通过排除 `@InitBinder` 等注解的方式来执行某些操作了。
相关推荐
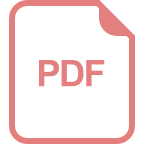
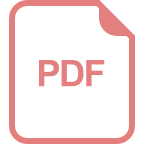














