使用python写一个函数,接受一个图像对图像进行二值化,高斯滤波,腐蚀最后对图片的最小外接圆轮廓截取,最后对截取下来的图像的敏感部分识别截取,最后对最终得到的部分中的数字进行识别,并输出
时间: 2023-06-25 08:06:13 浏览: 90
好的,下面是一个示例函数,可以实现你所需的功能。需要安装OpenCV和Tesseract OCR库。
```python
import cv2
import pytesseract
import numpy as np
def recognize_digits(image_path):
# 读取图像
img = cv2.imread(image_path)
# 转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 二值化
_, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)
# 高斯滤波
blur = cv2.GaussianBlur(thresh, (3, 3), 0)
# 腐蚀
kernel = np.ones((3, 3), np.uint8)
erosion = cv2.erode(blur, kernel, iterations=1)
# 查找最小外接圆轮廓
contours, _ = cv2.findContours(erosion, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
c = max(contours, key=cv2.contourArea)
(x, y), radius = cv2.minEnclosingCircle(c)
# 截取最小外接圆轮廓
x, y, radius = int(x), int(y), int(radius)
crop_img = img[y - radius:y + radius, x - radius:x + radius]
# 图像预处理
gray_crop = cv2.cvtColor(crop_img, cv2.COLOR_BGR2GRAY)
_, thresh_crop = cv2.threshold(gray_crop, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)
# 识别数字
digits = pytesseract.image_to_string(thresh_crop, config='--psm 10')
return digits
```
其中,`image_path`为输入的图像路径。函数会先将图像进行二值化、高斯滤波和腐蚀处理,然后查找最小外接圆轮廓并截取。最后使用Tesseract OCR来识别数字,并返回结果。
阅读全文
相关推荐
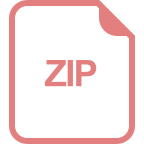
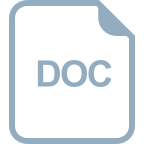
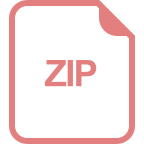
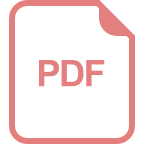
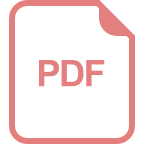
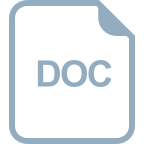
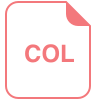
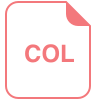
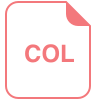
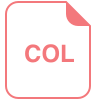
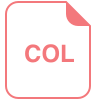
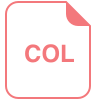
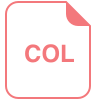
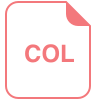
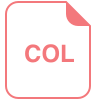
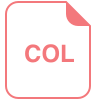
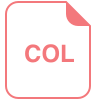
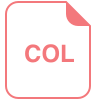