vue3中使用vxe table获取选中行
时间: 2023-11-26 08:03:06 浏览: 69
在 Vue3 中使用 VXE-Table 获取选中行,需要使用 `getCheckboxRecords` 方法,该方法可以获取当前表格中被勾选的所有行数据。具体实现步骤如下:
1. 在 Vue3 的 `setup` 函数中引入 VXE-Table 组件。
```javascript
import { ref } from 'vue'
import 'vxe-table/lib/style.css'
import VXETable from 'vxe-table'
export default {
setup() {
// 引入 VXE-Table 组件
VXETable.setup()
const tableData = ref([/* 表格数据 */])
const columns = ref([/* 表格列 */])
// ...
}
}
```
2. 在表格组件中添加 `checkbox-config` 属性,该属性可以配置表格中的多选框相关参数,如是否显示、选中列的绑定字段等。
```html
<template>
<vxe-table
:data="tableData"
:columns="columns"
:checkbox-config="{ showHeader: true, isAllSelected: false, checkField: 'isChecked' }"
/>
</template>
```
3. 在表格组件中添加获取选中行数据的方法,该方法可以在表格中勾选多选框后调用,获取当前被勾选的所有行数据。
```html
<template>
<vxe-table
:data="tableData"
:columns="columns"
:checkbox-config="{ showHeader: true, isAllSelected: false, checkField: 'isChecked' }"
ref="table"
/>
</template>
<script>
import { ref } from 'vue'
import 'vxe-table/lib/style.css'
import VXETable from 'vxe-table'
export default {
setup() {
// 引入 VXE-Table 组件
VXETable.setup()
const tableData = ref([/* 表格数据 */])
const columns = ref([/* 表格列 */])
// 获取表格组件的引用
const tableRef = ref(null)
// 获取选中行数据的方法
const getSelectedRows = () => {
return tableRef.value.getCheckboxRecords()
}
return {
tableData,
columns,
tableRef,
getSelectedRows
}
}
}
</script>
```
4. 在表格组件中调用获取选中行数据的方法,例如在点击按钮时获取选中行数据。
```html
<template>
<vxe-table
:data="tableData"
:columns="columns"
:checkbox-config="{ showHeader: true, isAllSelected: false, checkField: 'isChecked' }"
ref="table"
/>
<button @click="handleGetSelectedRows">获取选中行数据</button>
</template>
<script>
import { ref } from 'vue'
import 'vxe-table/lib/style.css'
import VXETable from 'vxe-table'
export default {
setup() {
// 引入 VXE-Table 组件
VXETable.setup()
const tableData = ref([/* 表格数据 */])
const columns = ref([/* 表格列 */])
// 获取表格组件的引用
const tableRef = ref(null)
// 获取选中行数据的方法
const getSelectedRows = () => {
return tableRef.value.getCheckboxRecords()
}
// 点击按钮时获取选中行数据
const handleGetSelectedRows = () => {
const selectedRows = getSelectedRows()
console.log(selectedRows)
}
return {
tableData,
columns,
tableRef,
getSelectedRows,
handleGetSelectedRows
}
}
}
</script>
```
相关推荐
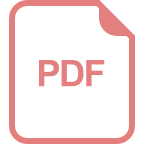
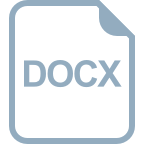
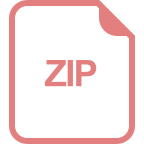














