根据你的回答给出布局文件和相关类
时间: 2023-10-20 17:05:24 浏览: 102
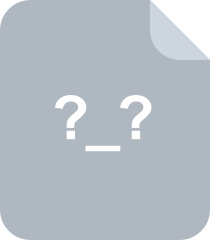
布局文件

好的,下面是一个简单的电话簿应用程序的布局文件和相关类。这里使用了 Android Studio 自带的模板和默认布局,你可以根据自己的需要进行修改和完善。
activity_main.xml:
```
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/main_layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="16dp"
tools:context=".MainActivity">
<TextView
android:id="@+id/title_textview"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="@string/title_text"
android:textAlignment="center"
android:textSize="24sp"
android:textStyle="bold" />
<ListView
android:id="@+id/contact_listview"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:divider="@null"
android:dividerHeight="0dp" />
<Button
android:id="@+id/add_button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="@string/add_button_text" />
</LinearLayout>
```
contact_item.xml:
```
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="8dp"
android:background="@color/colorPrimaryLight"
android:orientation="vertical"
android:padding="8dp">
<TextView
android:id="@+id/name_textview"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="18sp" />
<TextView
android:id="@+id/phone_textview"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="14sp" />
<TextView
android:id="@+id/other_info_textview"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="14sp" />
</LinearLayout>
```
Contact.java:
```
public class Contact {
private String name;
private String phone;
private String otherInfo;
public Contact(String name, String phone, String otherInfo) {
this.name = name;
this.phone = phone;
this.otherInfo = otherInfo;
}
public String getName() {
return name;
}
public String getPhone() {
return phone;
}
public String getOtherInfo() {
return otherInfo;
}
}
```
ContactAdapter.java:
```
public class ContactAdapter extends ArrayAdapter<Contact> {
private int resourceId;
public ContactAdapter(Context context, int resourceId, List<Contact> contacts) {
super(context, resourceId, contacts);
this.resourceId = resourceId;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
Contact contact = getItem(position);
View view;
ViewHolder viewHolder;
if (convertView == null) {
view = LayoutInflater.from(getContext()).inflate(resourceId, parent, false);
viewHolder = new ViewHolder();
viewHolder.nameTextView = (TextView) view.findViewById(R.id.name_textview);
viewHolder.phoneTextView = (TextView) view.findViewById(R.id.phone_textview);
viewHolder.otherInfoTextView = (TextView) view.findViewById(R.id.other_info_textview);
view.setTag(viewHolder);
} else {
view = convertView;
viewHolder = (ViewHolder) view.getTag();
}
viewHolder.nameTextView.setText(contact.getName());
viewHolder.phoneTextView.setText(contact.getPhone());
viewHolder.otherInfoTextView.setText(contact.getOtherInfo());
return view;
}
class ViewHolder {
TextView nameTextView;
TextView phoneTextView;
TextView otherInfoTextView;
}
}
```
MainActivity.java:
```
public class MainActivity extends AppCompatActivity {
private List<Contact> contactList = new ArrayList<>();
private ContactAdapter contactAdapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
initView();
}
private void initView() {
setTitle(R.string.app_name);
ListView contactListView = (ListView) findViewById(R.id.contact_listview);
contactAdapter = new ContactAdapter(this, R.layout.contact_item, contactList);
contactListView.setAdapter(contactAdapter);
Button addButton = (Button) findViewById(R.id.add_button);
addButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(MainActivity.this, AddContactActivity.class);
startActivityForResult(intent, 1);
}
});
contactListView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Contact contact = contactList.get(position);
AlertDialog.Builder builder = new AlertDialog.Builder(MainActivity.this);
builder.setTitle(R.string.delete_contact_title)
.setMessage(getString(R.string.delete_contact_message, contact.getName()))
.setPositiveButton(R.string.delete_button_text, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
contactList.remove(position);
contactAdapter.notifyDataSetChanged();
}
})
.setNegativeButton(R.string.cancel_button_text, null)
.show();
}
});
}
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == RESULT_OK && requestCode == 1) {
String name = data.getStringExtra("name");
String phone = data.getStringExtra("phone");
String otherInfo = data.getStringExtra("other_info");
Contact contact = new Contact(name, phone, otherInfo);
contactList.add(contact);
contactAdapter.notifyDataSetChanged();
}
}
}
```
AddContactActivity.java:
```
public class AddContactActivity extends AppCompatActivity {
private EditText nameEditText;
private EditText phoneEditText;
private EditText otherInfoEditText;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_add_contact);
initView();
}
private void initView() {
setTitle(R.string.add_contact_title);
nameEditText = (EditText) findViewById(R.id.name_edittext);
phoneEditText = (EditText) findViewById(R.id.phone_edittext);
otherInfoEditText = (EditText) findViewById(R.id.other_info_edittext);
Button saveButton = (Button) findViewById(R.id.save_button);
saveButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String name = nameEditText.getText().toString().trim();
String phone = phoneEditText.getText().toString().trim();
String otherInfo = otherInfoEditText.getText().toString().trim();
if (TextUtils.isEmpty(name) || TextUtils.isEmpty(phone)) {
Toast.makeText(AddContactActivity.this, R.string.empty_input_tip, Toast.LENGTH_SHORT).show();
} else {
Intent intent = new Intent();
intent.putExtra("name", name);
intent.putExtra("phone", phone);
intent.putExtra("other_info", otherInfo);
setResult(RESULT_OK, intent);
finish();
}
}
});
}
}
```
这些代码可以实现一个基本的电话簿应用程序,你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
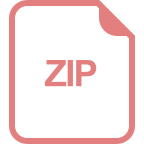
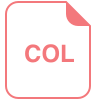














