js 类继承多个属性并添加自己的内容
时间: 2024-05-11 18:17:50 浏览: 125
在 JavaScript 中,可以使用 `extends` 关键字来实现类的继承。如果要继承多个父类的属性,可以使用 Mixin 的方式来实现。
Mixin 是一种将多个对象合并成一个对象的技术。在 JavaScript 中,可以使用 Object.assign() 方法将多个对象合并成一个新对象。我们可以将父类的属性通过 Object.assign() 方法合并到子类中。
下面是一个使用 Mixin 继承多个属性并添加自己内容的示例:
```js
class Dog {
constructor(name) {
this.name = name;
}
bark() {
console.log(`${this.name} is barking!`);
}
}
class Cat {
constructor(name) {
this.name = name;
}
meow() {
console.log(`${this.name} is meowing!`);
}
}
class Animal {
constructor(name) {
this.name = name;
}
eat() {
console.log(`${this.name} is eating!`);
}
}
// Mixin
const withJump = {
jump() {
console.log(`${this.name} is jumping!`);
}
};
class DogCat extends Animal {
constructor(name) {
super(name);
Object.assign(this, new Dog(name), new Cat(name), withJump);
}
play() {
console.log(`${this.name} is playing!`);
}
}
const dogCat = new DogCat('Tom');
dogCat.bark(); // Tom is barking!
dogCat.meow(); // Tom is meowing!
dogCat.jump(); // Tom is jumping!
dogCat.eat(); // Tom is eating!
dogCat.play(); // Tom is playing!
```
在上面的示例中,我们定义了一个 Dog 类和一个 Cat 类,并使用 Mixin 合并了它们的属性到 DogCat 类中。同时,我们还定义了一个 Animal 类,让 DogCat 类继承它的属性。最后,我们定义了一个 withJump 对象,它包含了 jump() 方法,将它也合并到 DogCat 类中。最终,我们创建了一个 DogCat 实例 `dogCat`,它同时具有了 Dog、Cat、Animal 和 withJump 的属性和方法。
阅读全文
相关推荐
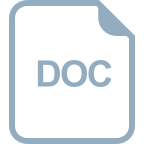
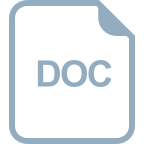
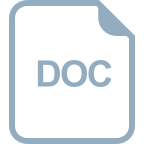
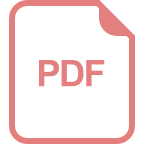
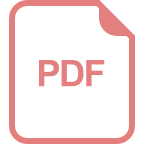
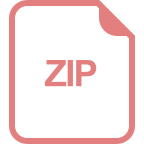
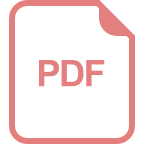
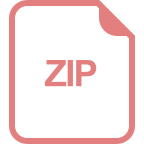
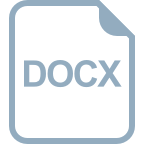
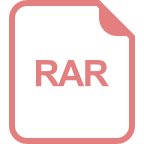
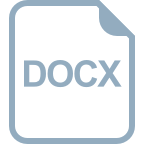
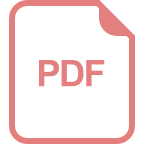
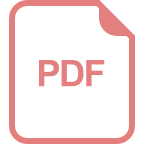
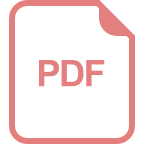
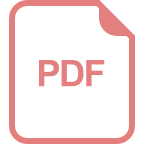
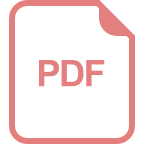
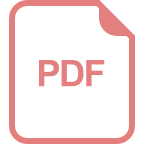
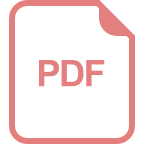
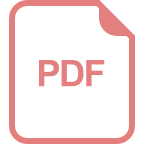