c# 多线程 List 增删改查
时间: 2023-07-05 16:36:13 浏览: 60
在 C# 中使用多线程访问 List 时,需要注意以下几点:
1. 多线程访问 List 时,可能会导致数据竞争,因此需要使用线程同步技术来保证访问的安全性。
2. 在多线程环境下,对 List 进行增删改查操作时,需要使用线程安全的集合类,例如 ConcurrentBag、ConcurrentDictionary、ConcurrentQueue、ConcurrentStack 等。
3. 如果需要自定义数据结构,可以使用 lock 关键字或者 Monitor 类来实现线程同步。
以下是一个简单的示例代码,用于演示在多线程环境下对 List 进行增删改查操作的方法:
```csharp
using System;
using System.Collections.Generic;
using System.Threading.Tasks;
class Program
{
static List<int> list = new List<int>();
static object locker = new object();
static void Main(string[] args)
{
// 使用 Parallel.For 来并行地向 List 中添加元素
Parallel.For(0, 10000, i =>
{
lock (locker)
{
list.Add(i);
}
});
// 使用 Parallel.ForEach 来并行地遍历 List
Parallel.ForEach(list, item =>
{
Console.WriteLine(item);
});
// 使用 Parallel.For 来并行地修改 List 中的元素
Parallel.For(0, list.Count, i =>
{
lock (locker)
{
list[i] = list[i] * 2;
}
});
// 使用 Parallel.For 来并行地删除 List 中的元素
Parallel.For(0, list.Count, i =>
{
lock (locker)
{
list.RemoveAt(i);
}
});
}
}
```
相关推荐
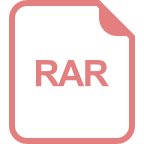














