@Override public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object arg2) throws Exception { // 该方法是在控制器方法前执行,如果为真,则继续下一步的操作,也就是执行控制器方法或调用下一个拦截器;为假,则中断 String uri=request.getRequestURI(); if(uri.indexOf("login")>0) { return true; } if(request.getSession().getAttribute("user")!=null) { return true; } // request.getSession().setAttribute("msg","您还没有登录,请先登录!"); request.getRequestDispatcher("/WEB-INF/jsp/login.jsp").forward(request, response); return false; }
时间: 2024-04-21 17:25:19 浏览: 17
根据你提供的代码可以看出,这是一个拦截器类的代码,用于拦截请求并进行处理。该拦截器的作用是检查用户是否已经登录,如果没有登录,则重定向到登录页面。如果已经登录,则放行请求。
该拦截器的核心代码是preHandle()方法,该方法在控制器方法前执行。在该方法中,首先获取请求的URI,然后判断该URI是否包含"login"字符串,如果包含,则放行请求,否则检查用户是否已经登录,如果已经登录,则放行请求,否则将请求转发到登录页面。
如果你的访问被拦截了,可以检查该拦截器的配置是否正确,或者检查你的请求是否符合该拦截器的拦截规则。如果你仍然无法解决问题,可以查看服务器的日志,查找错误信息,或者向开发人员寻求帮助。
相关问题
@Override public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler)
这是一个Java Web开发中的拦截器方法,用于在请求到达controller之前进行拦截处理。其中,HttpServletRequest代表HTTP请求,HttpServletResponse代表HTTP响应,Object handler代表请求的处理器。该方法返回一个boolean值,表示请求是否继续向下执行。如果返回true,请求继续向下执行;如果返回false,请求被拦截。
@Slf4j public class LoopCallInterceptor implements HandlerInterceptor { @Override public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception { //调用接口 // 头部获取当前请求所经过且没结束请求的path列表 // 判定path列表中是否包含当前path //无则正常访问 并记录到列表中 //有则告警循环调用,并终止调用,返回异常 return true; //return HandlerInterceptor.super.preHandle(request, response, handler); } @Override public void postHandle(HttpServletRequest request, HttpServletResponse response, Object handler, ModelAndView modelAndView) throws Exception { //HandlerInterceptor.super.postHandle(request, response, handler, modelAndView); //正常结束接口 //获取头部path列表 //从path列表中删除当前path } }
This is a Java class named `LoopCallInterceptor` that implements the `HandlerInterceptor` interface. The `HandlerInterceptor` interface is part of the Spring MVC framework and provides a way to intercept and handle requests and responses for Spring MVC applications.
The purpose of the `LoopCallInterceptor` class is to prevent circular or recursive calls to a Spring MVC controller method, which can cause infinite loops and consume excessive resources.
The `preHandle` method is called before a request is handled by a controller method. It takes in the `HttpServletRequest` and `HttpServletResponse` objects, as well as the `Object` representing the handler method that will handle the request.
The `preHandle` method first checks if the current request path is already present in the request header path list. If it is not present, it adds the current request path to the path list and returns `true` to indicate that the request can proceed normally. If it is already present, it logs a warning message for the circular or recursive call and returns `false` to indicate that the request should be stopped.
The `postHandle` method is called after a request has been handled by a controller method. It takes in the `HttpServletRequest`, `HttpServletResponse`, `Object` representing the handler method, and the `ModelAndView` object that contains the view and model data returned by the handler method.
The `postHandle` method removes the current request path from the request header path list.
Note that the `HandlerInterceptor` interface has three methods, but the `LoopCallInterceptor` class only implements the `preHandle` and `postHandle` methods. The `afterCompletion` method is called after the response has been rendered, but before the response has been committed.
相关推荐
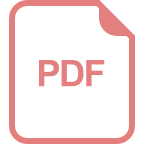
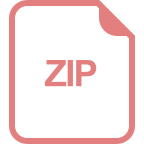












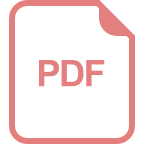