linux c语言实现base64加密
时间: 2023-07-28 17:34:09 浏览: 132
以下是一个简单的Linux C语言实现Base64加密的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
static const char base64_chars[] = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/";
char *base64_encode(const unsigned char *data, size_t input_length, size_t *output_length) {
*output_length = 4 * ((input_length + 2) / 3);
char *encoded_data = malloc(*output_length + 1);
if (encoded_data == NULL) return NULL;
for (size_t i = 0, j = 0; i < input_length;) {
uint32_t octet_a = i < input_length ? (unsigned char)data[i++] : 0;
uint32_t octet_b = i < input_length ? (unsigned char)data[i++] : 0;
uint32_t octet_c = i < input_length ? (unsigned char)data[i++] : 0;
uint32_t triple = (octet_a << 0x10) + (octet_b << 0x08) + octet_c;
encoded_data[j++] = base64_chars[(triple >> 3 * 6) & 0x3F];
encoded_data[j++] = base64_chars[(triple >> 2 * 6) & 0x3F];
encoded_data[j++] = base64_chars[(triple >> 1 * 6) & 0x3F];
encoded_data[j++] = base64_chars[(triple >> 0 * 6) & 0x3F];
}
for (size_t i = 0; i < (*output_length % 4); i++) {
encoded_data[*output_length - 1 - i] = '=';
}
return encoded_data;
}
int main(int argc, char *argv[]) {
const char *input_str = "hello, world!";
size_t input_len = strlen(input_str);
size_t output_len = 0;
char *encoded_str = base64_encode((const unsigned char *)input_str, input_len, &output_len);
printf("Encoded string: %s\n", encoded_str);
free(encoded_str);
return 0;
}
```
这个示例代码使用了一个包含64个字符的字符表(base64_chars),以及一个base64_encode函数,该函数将输入的数据编码成Base64格式,并返回编码后的字符串。在main函数中,我们首先声明一个字符串,然后计算它的长度,并将其传递给base64_encode函数。该函数将返回一个指向编码后的字符串的指针,我们可以将其打印出来。最后,我们释放了分配给编码字符串的内存。
阅读全文
相关推荐
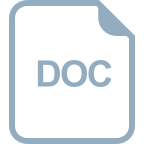
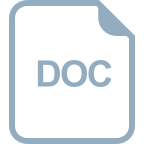
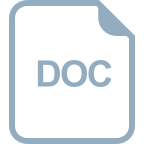

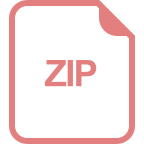

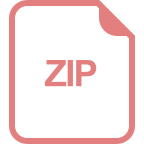
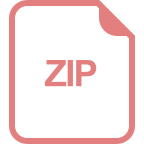
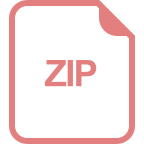
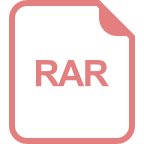
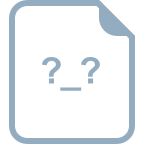
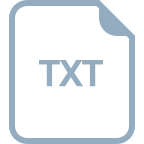
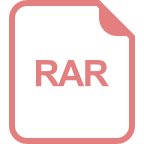
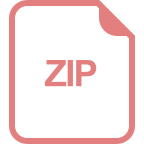
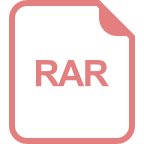
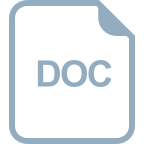
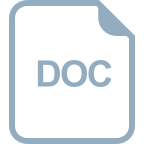
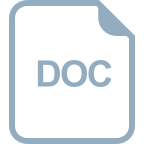
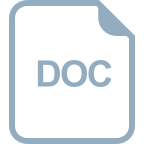