请用node和mysql帮我写一个根据用户借阅表里的借阅图书记录,然后再从图书表里选出图书推荐给用户的协同过滤推荐算法
时间: 2023-12-12 18:04:50 浏览: 32
好的,我可以为您编写一个简单的 Node.js 应用程序,它将根据用户借阅表中的记录执行协同过滤算法,然后从图书表中选择图书并向用户推荐。
首先,您需要确保已经安装了 Node.js 和 MySQL。然后,您需要创建两个表:一个是用户借阅表,另一个是图书表。用户借阅表应该至少包含以下列:user_id, book_id, borrow_date, return_date。图书表应该至少包含以下列:book_id, title, author。
在您的 Node.js 应用程序中,您可以使用以下代码来连接到 MySQL 数据库:
```javascript
const mysql = require('mysql');
const connection = mysql.createConnection({
host: 'localhost',
user: 'your_username',
password: 'your_password',
database: 'your_database'
});
connection.connect((err) => {
if (err) throw err;
console.log('Connected to MySQL database!');
});
```
然后,您可以使用以下代码从用户借阅表中检索数据:
```javascript
const userId = 1; // replace with actual user ID
const borrowQuery = `SELECT book_id FROM borrow_table WHERE user_id = ${userId}`;
connection.query(borrowQuery, (err, results, fields) => {
if (err) throw err;
const bookIds = results.map(result => result.book_id);
// perform collaborative filtering algorithm here
// recommend books to user
});
```
接下来,您需要实现协同过滤算法。这可能需要一些复杂的数学计算,但以下是一个简单的示例,它使用用户借阅表中的数据来查找与当前用户最相似的其他用户,并从他们的借阅记录中选择推荐的书籍:
```javascript
const borrowCounts = {};
// count the number of times each book has been borrowed by all users
bookIds.forEach(bookId => {
const countQuery = `SELECT COUNT(*) AS borrow_count FROM borrow_table WHERE book_id = ${bookId}`;
connection.query(countQuery, (err, results, fields) => {
if (err) throw err;
const borrowCount = results[0].borrow_count;
borrowCounts[bookId] = borrowCount;
});
});
// find the most similar user by comparing their borrow history
const similarityScores = {};
const similarityQuery = `SELECT user_id FROM borrow_table WHERE book_id IN (${bookIds.join(',')}) AND user_id != ${userId}`;
connection.query(similarityQuery, (err, results, fields) => {
if (err) throw err;
results.forEach(result => {
const otherUserId = result.user_id;
const otherUserBorrowQuery = `SELECT book_id FROM borrow_table WHERE user_id = ${otherUserId}`;
connection.query(otherUserBorrowQuery, (err, borrowResults, fields) => {
if (err) throw err;
const otherUserBookIds = borrowResults.map(result => result.book_id);
const similarity = otherUserBookIds.filter(bookId => bookIds.includes(bookId)).length / bookIds.length;
similarityScores[otherUserId] = similarity;
});
});
const mostSimilarUser = Object.keys(similarityScores).reduce((a, b) => similarityScores[a] > similarityScores[b] ? a : b);
// recommend books based on most similar user's borrowing history
const recommendQuery = `SELECT book_id FROM borrow_table WHERE user_id = ${mostSimilarUser} ORDER BY borrow_date DESC LIMIT 5`;
connection.query(recommendQuery, (err, results, fields) => {
if (err) throw err;
const recommendedBookIds = results.map(result => result.book_id);
const recommendedBooks = recommendedBookIds.map(bookId => {
const bookQuery = `SELECT title, author FROM book_table WHERE book_id = ${bookId}`;
connection.query(bookQuery, (err, bookResults, fields) => {
if (err) throw err;
const book = bookResults[0];
return { id: bookId, title: book.title, author: book.author, borrow_count: borrowCounts[bookId] };
});
});
// sort recommended books by the number of times they have been borrowed
recommendedBooks.sort((a, b) => b.borrow_count - a.borrow_count);
// recommend books to user
});
});
```
最后,您需要将推荐的图书列表返回给用户。您可以将它们作为 JSON 格式的数组发送到客户端,或使用模板引擎将它们呈现为 HTML 页面。
这只是一个简单的示例,实际的协同过滤算法可能会涉及更复杂的数学计算和更多的优化。但希望这可以为您提供一个起点,以便开始构建您自己的推荐系统。
相关推荐
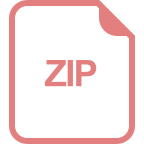
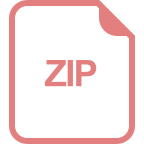
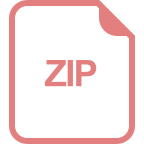














