c语言小游戏
时间: 2023-06-22 10:17:33 浏览: 199
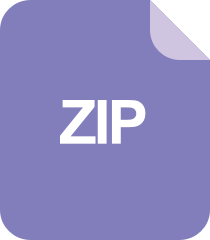
C语言三款经典代码游戏
好的,我可以为您提供一些简单的C语言小游戏的示例代码,您可以参考或者修改它们来满足您的需求。
1. 猜数字游戏
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main()
{
int num, guess, count = 0;
srand(time(0)); // 初始化随机数种子
num = rand() % 100 + 1; // 生成1~100之间的随机数
printf("猜数字游戏!\n");
do {
printf("请输入一个1~100之间的整数:");
scanf("%d", &guess);
count++;
if (guess > num) {
printf("猜大了!\n");
} else if (guess < num) {
printf("猜小了!\n");
} else {
printf("恭喜你,猜对了!\n");
printf("你一共猜了%d次。\n", count);
}
} while (guess != num);
return 0;
}
```
2. 井字棋游戏
```c
#include <stdio.h>
int main()
{
char board[3][3] = {{' ', ' ', ' '}, {' ', ' ', ' '}, {' ', ' ', ' '}};
int row, col, player = 1;
printf("井字棋游戏!\n");
while (1) {
// 打印当前棋盘
printf("\n");
printf(" %c | %c | %c \n", board[0][0], board[0][1], board[0][2]);
printf("---+---+---\n");
printf(" %c | %c | %c \n", board[1][0], board[1][1], board[1][2]);
printf("---+---+---\n");
printf(" %c | %c | %c \n", board[2][0], board[2][1], board[2][2]);
// 判断胜负
if (board[0][0] == 'X' && board[0][1] == 'X' && board[0][2] == 'X'
|| board[1][0] == 'X' && board[1][1] == 'X' && board[1][2] == 'X'
|| board[2][0] == 'X' && board[2][1] == 'X' && board[2][2] == 'X'
|| board[0][0] == 'X' && board[1][0] == 'X' && board[2][0] == 'X'
|| board[0][1] == 'X' && board[1][1] == 'X' && board[2][1] == 'X'
|| board[0][2] == 'X' && board[1][2] == 'X' && board[2][2] == 'X'
|| board[0][0] == 'X' && board[1][1] == 'X' && board[2][2] == 'X'
|| board[0][2] == 'X' && board[1][1] == 'X' && board[2][0] == 'X') {
printf("恭喜玩家1获胜!\n");
break;
} else if (board[0][0] == 'O' && board[0][1] == 'O' && board[0][2] == 'O'
|| board[1][0] == 'O' && board[1][1] == 'O' && board[1][2] == 'O'
|| board[2][0] == 'O' && board[2][1] == 'O' && board[2][2] == 'O'
|| board[0][0] == 'O' && board[1][0] == 'O' && board[2][0] == 'O'
|| board[0][1] == 'O' && board[1][1] == 'O' && board[2][1] == 'O'
|| board[0][2] == 'O' && board[1][2] == 'O' && board[2][2] == 'O'
|| board[0][0] == 'O' && board[1][1] == 'O' && board[2][2] == 'O'
|| board[0][2] == 'O' && board[1][1] == 'O' && board[2][0] == 'O') {
printf("恭喜玩家2获胜!\n");
break;
} else if (board[0][0] != ' ' && board[0][1] != ' ' && board[0][2] != ' '
&& board[1][0] != ' ' && board[1][1] != ' ' && board[1][2] != ' '
&& board[2][0] != ' ' && board[2][1] != ' ' && board[2][2] != ' ') {
printf("平局!\n");
break;
}
// 下棋
if (player == 1) {
printf("玩家1,请输入行列坐标(例如:1 2):");
} else {
printf("玩家2,请输入行列坐标(例如:1 2):");
}
scanf("%d %d", &row, &col);
if (row < 1 || row > 3 || col < 1 || col > 3) {
printf("无效的坐标,请重新输入!\n");
continue;
}
if (board[row - 1][col - 1] != ' ') {
printf("该位置已经有棋子了,请重新输入!\n");
continue;
}
if (player == 1) {
board[row - 1][col - 1] = 'X';
player = 2;
} else {
board[row - 1][col - 1] = 'O';
player = 1;
}
}
return 0;
}
```
希望这些示例代码可以对您有所帮助!
阅读全文
相关推荐
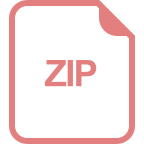
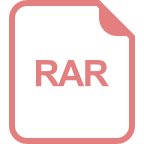
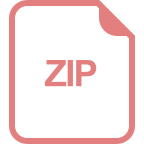
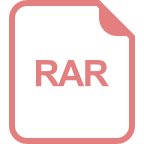
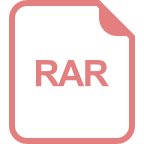
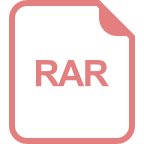
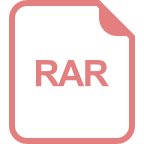
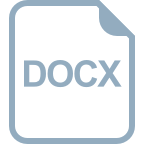
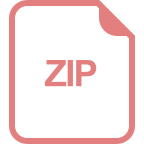
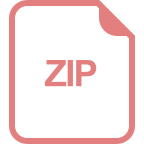
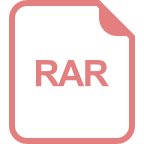
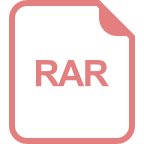