Qt 拷贝文件夹代码
时间: 2024-11-22 22:44:29 浏览: 5
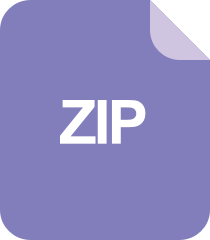
Qt文件或文件夹拷贝
在Qt中,你可以使用QDir和QFile类以及它们的相关函数来复制整个文件夹。以下是一个简单的示例,展示如何递归地复制一个源文件夹到目标文件夹:
```cpp
#include <QDir>
#include <QFile>
#include <QDebug>
void copyDirectory(const QString &sourcePath, const QString &targetPath)
{
QDir source(sourcePath);
if (!source.exists() || !source.isWritable()) {
qDebug() << "Source directory not found or not writable";
return;
}
QDir target(targetPath);
if (!target.mkpath(targetPath)) {
qDebug() << "Failed to create target directory";
return;
}
QStringList entries = source.entryList(QDir::Dirs | QDir::Files, QDir::AllEntries);
for (const QString &entry : entries) {
QString sourceFilePath = source.path() + "/" + entry;
QString targetFilePath = targetPath + "/" + entry;
QFile file(sourceFilePath);
if (file.exists()) {
if (file.isFile()) {
if (!file.copy(targetFilePath)) {
qDebug() << "Failed to copy file: " << targetFilePath;
}
} else {
copyDirectory(sourceFilePath, targetFilePath);
}
} else {
qDebug() << "Entry not found: " << sourceFilePath;
}
}
}
// 使用示例
int main(int argc, char *argv[])
{
QString srcDir = "/path/to/source/directory";
QString dstDir = "/path/to/destination/directory";
copyDirectory(srcDir, dstDir);
return QApplication::exec();
}
```
这个代码首先检查源目录是否存在且可写,然后创建目标目录(如果不存在)。接着遍历源目录中的所有文件和子目录,并递归处理子目录。如果遇到文件,则尝试复制;如果遇到子目录则继续复制。
阅读全文
相关推荐
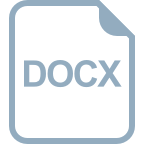
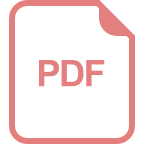















