java获取跨网段的mac地址
时间: 2023-10-18 14:04:37 浏览: 174
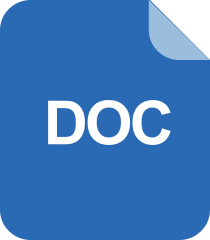
跨网段获取远程主机MAC地址的方法与java实现

要获取跨网段的MAC地址,你需要使用ARP协议。ARP协议需要发送广播消息来获取目标设备的MAC地址。下面是一个Java代码示例:
```java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.InetAddress;
import java.net.NetworkInterface;
import java.util.Collections;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class MacAddressGetter {
public static void main(String[] args) throws Exception {
// 目标IP地址
String ipAddress = "192.168.0.1";
// 获取本地网络接口列表
List<NetworkInterface> interfaces = Collections.list(NetworkInterface.getNetworkInterfaces());
// 循环遍历网络接口
for (NetworkInterface intf : interfaces) {
// 获取IP地址列表
List<InetAddress> addrs = Collections.list(intf.getInetAddresses());
for (InetAddress addr : addrs) {
// 判断是否为目标IP地址
if (addr.getHostAddress().equals(ipAddress)) {
// 获取MAC地址
byte[] mac = intf.getHardwareAddress();
System.out.println("MAC地址为:" + bytesToHex(mac));
return;
}
}
}
// 如果没有找到对应的网络接口,则发送ARP请求获取MAC地址
String command = "arp -a " + ipAddress;
Process process = Runtime.getRuntime().exec(command);
BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()));
String line;
Pattern pattern = Pattern.compile("([0-9A-Fa-f]{2}[:-]){5}([0-9A-Fa-f]{2})");
while ((line = reader.readLine()) != null) {
Matcher matcher = pattern.matcher(line);
if (matcher.find()) {
String macAddress = matcher.group();
System.out.println("MAC地址为:" + macAddress);
return;
}
}
}
/**
* 将字节数组转换为十六进制字符串
*/
public static String bytesToHex(byte[] bytes) {
StringBuilder builder = new StringBuilder();
for (byte b : bytes) {
builder.append(String.format("%02X", b));
builder.append(":");
}
builder.deleteCharAt(builder.length() - 1);
return builder.toString();
}
}
```
这段代码首先通过遍历本地网络接口列表来查找是否存在目标IP地址的网络接口,并获取该接口的MAC地址。如果没有找到,则发送ARP请求获取MAC地址。注意,这种方法只适用于局域网内的设备,因为ARP请求只能在同一网段内广播。如果需要跨越不同的网段获取MAC地址,需要使用其他方法,例如通过SNMP协议查询设备信息。
阅读全文
相关推荐
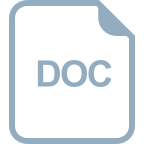
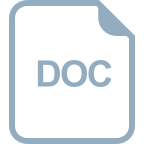

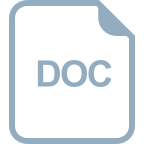


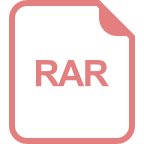
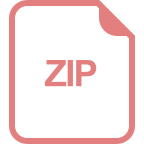
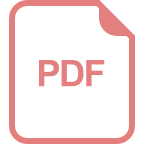
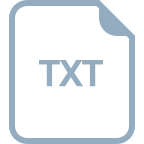
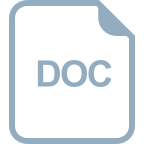
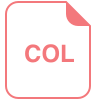
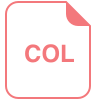
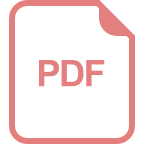
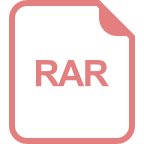
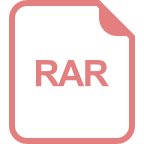
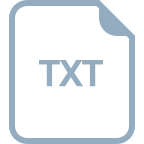
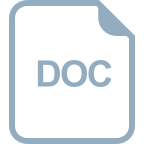