使用Pygame制作一个简单的球类游戏,实现游戏界面,游戏的得分机制
时间: 2024-03-05 12:54:29 浏览: 20
好的,我可以为您提供一些关于制作这个游戏的提示和建议。
首先,您需要安装Pygame库。如果您使用的是Anaconda,可以通过以下命令进行安装:
```
conda install -c cogsci pygame
```
接下来,您可以开始编写代码了。以下是一个基本框架,包括游戏窗口和球的移动:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 设置游戏窗口大小和标题
screen_width = 800
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("Ball Game")
# 定义球的属性
ball_radius = 20
ball_color = (255, 0, 0)
ball_x = random.randint(ball_radius, screen_width - ball_radius)
ball_y = random.randint(ball_radius, screen_height - ball_radius)
ball_speed_x = 5
ball_speed_y = 5
# 游戏主循环
while True:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# 移动球
ball_x += ball_speed_x
ball_y += ball_speed_y
# 碰撞检测
if ball_x < ball_radius or ball_x > screen_width - ball_radius:
ball_speed_x = -ball_speed_x
if ball_y < ball_radius or ball_y > screen_height - ball_radius:
ball_speed_y = -ball_speed_y
# 绘制游戏界面
screen.fill((255, 255, 255))
pygame.draw.circle(screen, ball_color, (ball_x, ball_y), ball_radius)
pygame.display.update()
```
这个代码会创建一个游戏窗口,并在窗口中绘制一个红色的球。球会以每秒5像素的速度移动,如果碰到窗口边缘,会反弹。
现在,您可以通过添加一些游戏逻辑来实现得分机制。例如,您可以添加一个分数变量,并在球碰到窗口边缘时增加分数。这里是一个示例代码:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 设置游戏窗口大小和标题
screen_width = 800
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("Ball Game")
# 定义球的属性
ball_radius = 20
ball_color = (255, 0, 0)
ball_x = random.randint(ball_radius, screen_width - ball_radius)
ball_y = random.randint(ball_radius, screen_height - ball_radius)
ball_speed_x = 5
ball_speed_y = 5
# 定义分数
score = 0
font = pygame.font.Font(None, 36)
# 游戏主循环
while True:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# 移动球
ball_x += ball_speed_x
ball_y += ball_speed_y
# 碰撞检测
if ball_x < ball_radius or ball_x > screen_width - ball_radius:
ball_speed_x = -ball_speed_x
score += 1
if ball_y < ball_radius or ball_y > screen_height - ball_radius:
ball_speed_y = -ball_speed_y
score += 1
# 绘制游戏界面
screen.fill((255, 255, 255))
pygame.draw.circle(screen, ball_color, (ball_x, ball_y), ball_radius)
# 绘制分数
score_text = font.render("Score: {}".format(score), True, (0, 0, 0))
screen.blit(score_text, (10, 10))
pygame.display.update()
```
这个代码会在窗口左上角显示一个分数,并在球碰到窗口边缘时增加分数。您可以根据自己的需求修改代码,并添加其他游戏元素,例如障碍物、加速道具等等。
相关推荐
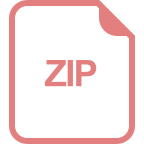
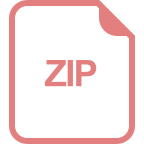














