kmeans聚类算法matlab python
时间: 2023-08-31 18:05:21 浏览: 114
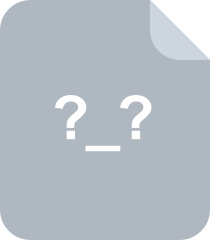
kmeans聚类算法
K-means 聚类算法是一种常用的无监督学习算法,可以对数据进行聚类分析。它的基本思想是将样本数据划分为 K 个簇,使得簇内的数据点尽量相似,而簇间的数据点相互之间尽量不相似。下面分别介绍在 MATLAB 和 Python 中如何实现 K-means 聚类算法。
MATLAB 实现:
MATLAB 中可以使用自带的 kmeans 函数来实现 K-means 聚类算法。函数的调用格式为:
[idx, C] = kmeans(X, K)
其中,X 是一个 n 行 d 列的矩阵,表示 n 个样本数据,每个样本有 d 个特征;K 表示要将数据分成的簇的个数;idx 是一个 n 行 1 列的向量,表示每个样本所属的簇的编号;C 是一个 K 行 d 列的矩阵,表示每个簇的中心点的坐标。
下面是一个 MATLAB 示例代码:
```matlab
% 生成数据
rng('default');
X = [randn(100,2)*0.75+ones(100,2);
randn(100,2)*0.5-ones(100,2)];
% 调用 kmeans 函数进行聚类
K = 2;
[idx, C] = kmeans(X, K);
% 可视化结果
figure;
scatter(X(idx==1,1), X(idx==1,2), 'r');
hold on;
scatter(X(idx==2,1), X(idx==2,2), 'b');
scatter(C(:,1), C(:,2), 'k', 'filled');
legend('Cluster 1', 'Cluster 2', 'Centroids');
```
Python 实现:
Python 中可以使用 scikit-learn 库来实现 K-means 聚类算法。具体可以使用 KMeans 类来实现,代码如下:
```python
from sklearn.cluster import KMeans
import numpy as np
import matplotlib.pyplot as plt
# 生成数据
np.random.seed(0)
X = np.r_[np.random.randn(100,2)*0.75 + np.ones((100,2)),
np.random.randn(100,2)*0.5 - np.ones((100,2))]
# 调用 KMeans 类进行聚类
kmeans = KMeans(n_clusters=2, random_state=0).fit(X)
labels = kmeans.labels_
centers = kmeans.cluster_centers_
# 可视化结果
plt.scatter(X[labels==0,0], X[labels==0,1], c='r')
plt.scatter(X[labels==1,0], X[labels==1,1], c='b')
plt.scatter(centers[:,0], centers[:,1], marker='x', s=200, linewidths=3, color='k')
plt.legend(['Cluster 1', 'Cluster 2', 'Centroids'])
plt.show()
```
以上是在 MATLAB 和 Python 中实现 K-means 聚类算法的示例代码,可以根据自己的数据和需求进行调整。
阅读全文
相关推荐
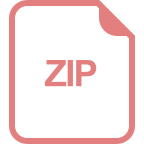
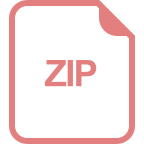
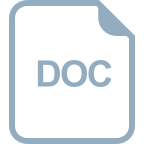
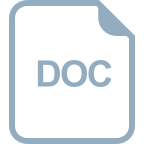

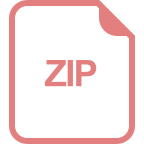
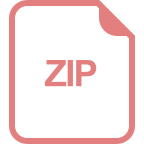
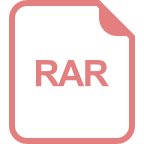
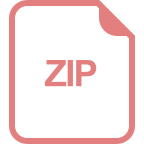
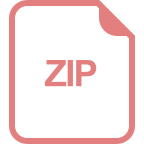
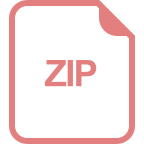
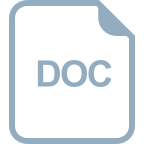
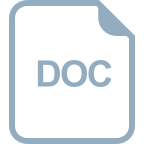
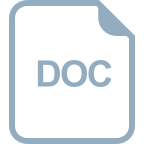
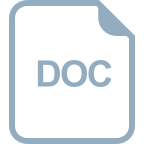

