public List<EquipmentDO> getExpiringEquipments(LocalDateTime now, LocalDateTime threeMonthsLater) { //TODO:返回DOlist,当过期时间在 between now and threemonths 的 DO return null; } 帮忙完善该方法以及Mapper
时间: 2024-04-12 16:31:03 浏览: 17
好的,下面是一个可能的实现方案:
1. 首先你需要确保你的`EquipmentDO`类中有一个表示过期时间的字段。假设这个字段叫做`expiryDate`,是一个`LocalDateTime`类型的变量。
2. 在Mapper接口中,你需要定义一个方法来查询过期的设备。假设你的Mapper接口叫做`EquipmentMapper`,可以在其中添加如下方法:
```java
public interface EquipmentMapper {
List<EquipmentDO> getExpiringEquipments(@Param("now") LocalDateTime now, @Param("threeMonthsLater") LocalDateTime threeMonthsLater);
}
```
3. 然后,在对应的Mapper XML文件中,你可以编写相应的SQL查询语句来获取过期的设备。假设你的Mapper XML文件叫做`EquipmentMapper.xml`,可以在其中添加如下代码:
```xml
<mapper namespace="com.example.mapper.EquipmentMapper">
<select id="getExpiringEquipments" resultType="com.example.model.EquipmentDO">
SELECT * FROM equipment
WHERE expiry_date BETWEEN #{now} AND #{threeMonthsLater}
</select>
</mapper>
```
请确保将上述代码中的表名、字段名和命名空间等根据实际情况进行替换。
4. 最后,在你的服务类或者控制器中使用该方法来获取过期设备。假设你的服务类叫做`EquipmentService`,可以在其中注入`EquipmentMapper`并调用该方法:
```java
@Service
public class EquipmentService {
private final EquipmentMapper equipmentMapper;
public EquipmentService(EquipmentMapper equipmentMapper) {
this.equipmentMapper = equipmentMapper;
}
public List<EquipmentDO> getExpiringEquipments(LocalDateTime now, LocalDateTime threeMonthsLater) {
return equipmentMapper.getExpiringEquipments(now, threeMonthsLater);
}
}
```
请确保将上述代码中的包名和类名根据实际情况进行替换。
这样,当你调用`getExpiringEquipments`方法并传入当前时间和三个月后的时间,就会返回在这个时间范围内过期的设备列表。
希望这个实现方案能对你有所帮助!如果你还有其他问题,请随时提问。
相关推荐
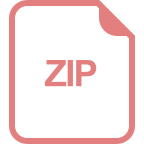
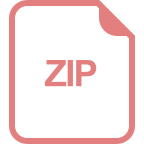
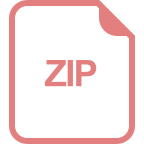














