编写程序:定义一个抽象类Shape,其中包含两个纯虚函数GetArea()和GetPerimeter()。以Shape类作为基类创建Circle类和Rectangle类,这两个类对Shape类中的两个纯虚函数进行了重定义。Circle类中的GetArea()和GetPerimeter()函数分别用来计算圆的面积和周长;Rectangle类中的GetArea()和GetPerimeter()函数分别用来计算矩形的面积和周长。例如,执行主程序,可以在屏幕上输出:半径为3的圆的面积和周长、长和宽分别为5和8的矩
时间: 2024-03-22 22:39:32 浏览: 82
下面是程序的实现:
```c++
#include <iostream>
using namespace std;
class Shape {
public:
virtual double GetArea() = 0;
virtual double GetPerimeter() = 0;
};
class Circle : public Shape {
private:
double m_radius;
public:
Circle(double radius) {
m_radius = radius;
}
double GetArea() {
return 3.14159 * m_radius * m_radius;
}
double GetPerimeter() {
return 2 * 3.14159 * m_radius;
}
};
class Rectangle : public Shape {
private:
double m_length;
double m_width;
public:
Rectangle(double length, double width) {
m_length = length;
m_width = width;
}
double GetArea() {
return m_length * m_width;
}
double GetPerimeter() {
return 2 * (m_length + m_width);
}
};
int main() {
Circle circle(3);
Rectangle rectangle(5, 8);
cout << "半径为3的圆的面积为:" << circle.GetArea() << endl;
cout << "半径为3的圆的周长为:" << circle.GetPerimeter() << endl;
cout << "长为5宽为8的矩形的面积为:" << rectangle.GetArea() << endl;
cout << "长为5宽为8的矩形的周长为:" << rectangle.GetPerimeter() << endl;
return 0;
}
```
在这个程序中,我们定义了一个抽象类 Shape,其中包含了两个纯虚函数 GetArea 和 GetPerimeter。然后,我们又定义了一个 Circle 类和一个 Rectangle 类,它们都继承自 Shape 类,并重定义了 Shape 类中的两个纯虚函数。
在 Circle 类中,我们新增了一个半径成员变量,然后在 GetArea 和 GetPerimeter 函数中分别计算圆的面积和周长,并返回计算结果。
在 Rectangle 类中,我们新增了长和宽两个成员变量,然后在 GetArea 和 GetPerimeter 函数中分别计算矩形的面积和周长,并返回计算结果。
最后,在主函数中,我们分别创建了一个 Circle 类的对象和一个 Rectangle 类的对象,并调用它们的 GetArea 和 GetPerimeter 函数来计算圆的面积和周长,以及矩形的面积和周长,并输出计算结果。
阅读全文
相关推荐
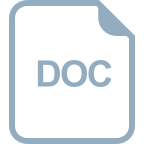
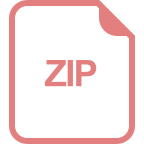
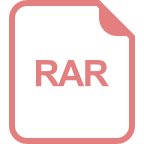




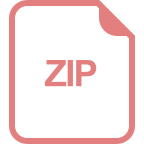
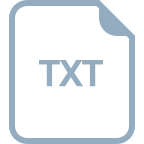








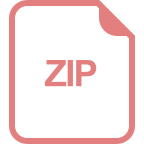
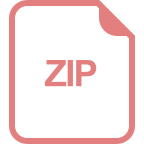