vue element tree 控制单选多选
时间: 2023-11-13 13:02:58 浏览: 114
Vue Element Tree 组件支持单选和多选的功能,你可以通过设置 `show-checkbox` 属性来控制是否显示复选框,然后使用 `default-checked-keys` 或 `default-selected-keys` 属性来设置默认选中的节点。
如果你希望让树组件支持多选,可以将 `show-checkbox` 属性设置为 `true`,然后在 `@check-change` 事件中监听复选框的选中状态,获取选中的节点信息。
如果你希望让树组件支持单选,可以将 `show-checkbox` 属性设置为 `false`,然后在 `@node-click` 事件中监听节点的点击事件,获取选中的节点信息,并使用 `current-node-key` 属性来控制当前选中的节点。
相关问题
vue2 el-tree实现单选
要在Vue 2中使用el-tree实现单选,你可以使用其check-strictly属性来限制仅允许选择一个节点。以下是一个简单的示例:
首先,在你的Vue组件中导入el-tree组件:
```javascript
import { Tree } from 'element-ui';
```
然后,在你的模板中使用el-tree组件,并设置check-strictly属性为true:
```html
<template>
<div>
<el-tree :data="treeData" :props="treeProps" show-checkbox check-strictly @check="handleCheck"></el-tree>
</div>
</template>
```
在Vue实例中,你需要定义treeData和treeProps:
```javascript
data() {
return {
treeData: [
{
id: 1,
label: 'Node 1',
children: [
{
id: 11,
label: 'Node 1-1'
},
{
id: 12,
label: 'Node 1-2'
}
]
},
{
id: 2,
label: 'Node 2'
}
],
treeProps: {
children: 'children',
label: 'label'
}
};
},
```
最后,实现handleCheck方法来处理选中节点的逻辑:
```javascript
methods: {
handleCheck(node) {
// 取消其他节点的选中状态
this.$refs.tree.setCheckedKeys([node.id]);
}
}
```
这样,当你选择一个节点时,其他节点就会自动取消选中状态,实现了单选效果。
希望这能帮到你!
vue3 el-tree 单选
在 Vue 3 中使用 el-tree 进行单选操作,你可以通过自定义节点模板来实现。下面是一个简单的示例:
首先,你需要在组件中引入 el-tree 和 el-radio 组件:
```javascript
import { ElTree, ElRadio } from 'element-plus';
```
然后,在模板中使用 el-tree 组件,并在节点模板中添加 el-radio 组件:
```html
<template>
<el-tree :data="treeData" :props="treeProps">
<template #default="{ node }">
<el-radio v-model="selectedNodeId" :label="node.id"></el-radio>
<span>{{ node.label }}</span>
</template>
</el-tree>
</template>
```
在上述代码中,我们使用了 `v-model` 指令来绑定选中节点的 `id` 到 `selectedNodeId` 变量。
接下来,你需要在组件的 `data` 中定义 `treeData` 和 `selectedNodeId` 变量:
```javascript
data() {
return {
treeData: [
{ id: 1, label: 'Node 1', children: [
{ id: 11, label: 'Node 1-1' },
{ id: 12, label: 'Node 1-2' },
]},
{ id: 2, label: 'Node 2', children: [
{ id: 21, label: 'Node 2-1' },
{ id: 22, label: 'Node 2-2' },
]}
],
selectedNodeId: null
};
}
```
最后,你可以根据 `selectedNodeId` 来获取选中的节点信息。例如,你可以添加一个计算属性来获取选中的节点对象:
```javascript
computed: {
selectedNode() {
return this.getNodeById(this.selectedNodeId);
}
},
methods: {
getNodeById(id, nodes = this.treeData) {
for (const node of nodes) {
if (node.id === id) {
return node;
}
if (node.children) {
const foundNode = this.getNodeById(id, node.children);
if (foundNode) {
return foundNode;
}
}
}
return null;
}
}
```
现在,你已经可以在 el-tree 中实现单选功能了。通过 `selectedNodeId` 变量,你可以获取选中的节点信息,并根据需求进行处理。
希望这个例子能帮助到你!如果还有其他问题,请随时提问。
阅读全文
相关推荐
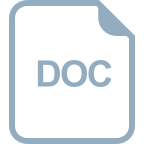
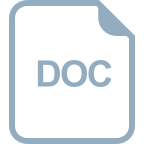
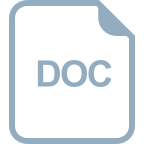
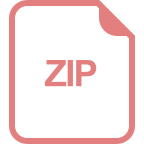
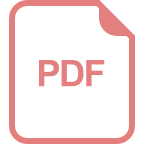
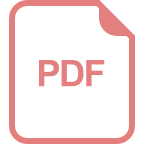
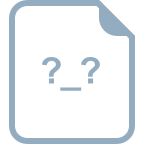
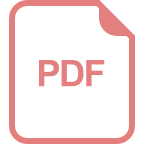







