SpringBoot写一个中转文件(附带参数信息)接口的详细代码例子并标识备注
时间: 2024-06-11 13:09:01 浏览: 141
以下是一个使用SpringBoot编写的中转文件接口的示例代码,包括注释以解释每个部分的作用。
```java
@RestController
public class FileTransferController {
@Autowired
private ResourceLoader resourceLoader;
@PostMapping("/transfer")
public ResponseEntity<Resource> transferFile(@RequestParam("file") MultipartFile file,
@RequestParam(value = "type", required = false) String fileType) {
// 获取文件名和类型
String fileName = file.getOriginalFilename();
String contentType = file.getContentType();
// 如果没有传递文件类型,则使用文件的实际类型
if (fileType == null) {
fileType = contentType;
}
// 将文件保存到服务器的临时目录中
try {
File tempFile = File.createTempFile("temp", null);
file.transferTo(tempFile);
// 将文件返回给客户端
Resource resource = resourceLoader.getResource("file:" + tempFile.getAbsolutePath());
return ResponseEntity.ok()
.contentType(MediaType.parseMediaType(fileType))
.contentLength(resource.contentLength())
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + fileName + "\"")
.body(resource);
} catch (IOException e) {
e.printStackTrace();
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build();
}
}
}
```
这个控制器类有一个`transferFile`方法,这个方法接收一个`MultipartFile`类型的文件和一个可选的`type`参数。如果提供了`type`参数,则将其用作文件的MIME类型,否则使用文件的实际MIME类型。
然后,该方法将文件保存到服务器的临时目录中,并使用Spring的`ResourceLoader`来获取文件的资源对象。最后,该方法将资源对象包装在一个`ResponseEntity`中,并将其返回给客户端。客户端可以使用此响应打开或下载文件。
此示例中的中转文件接口可以通过POST方法调用,如下所示:
```
POST /transfer HTTP/1.1
Host: example.com
Content-Type: multipart/form-data; boundary=----WebKitFormBoundary7MA4YWxkTrZu0gW
Content-Disposition: form-data; name="file"; filename="example.pdf"
Content-Type: application/pdf
<@INCLUDE *path-to-file*/example.pdf>
------WebKitFormBoundary7MA4YWxkTrZu0gW
Content-Disposition: form-data; name="type"
application/pdf
------WebKitFormBoundary7MA4YWxkTrZu0gW--
```
在这个示例中,我们使用了一个名为`file`的表单字段来上传文件,并在另一个名为`type`的字段中提供了可选的文件类型。此请求将上传一个名为`example.pdf`的PDF文件,并将其保存在服务器上的临时目录中。服务器将返回一个包含`example.pdf`的PDF文件的`ResponseEntity`。
阅读全文
相关推荐
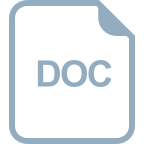
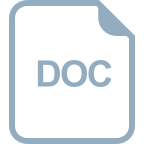
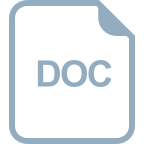
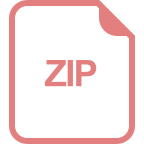
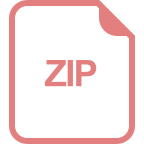
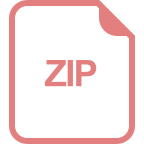
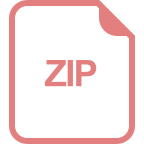
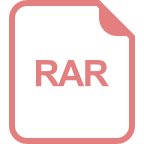
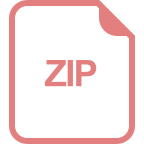
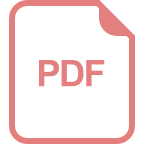
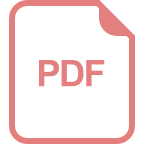
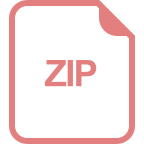
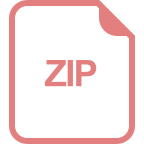
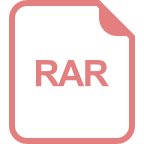