c语言 获取多个日期文件夹内的文件数量和名字,并且通过结构体保存下来
时间: 2024-01-20 09:02:57 浏览: 63
可以通过以下步骤来实现获取多个日期文件夹内的文件数量和名字,并且通过结构体保存下来:
1. 定义一个结构体来保存文件名和数量:
```
typedef struct {
char filename[100];
int count;
} FileInfo;
```
2. 编写一个函数来获取文件夹内的文件数量和名字:
```
int get_file_info(char *folder_path, FileInfo *file_info) {
int file_count = 0;
DIR *dir;
struct dirent *entry;
dir = opendir(folder_path);
if (dir == NULL) {
perror("opendir error");
return -1;
}
while ((entry = readdir(dir)) != NULL) {
if (entry->d_type == DT_REG) {
strcpy(file_info[file_count].filename, entry->d_name);
file_info[file_count].count = 0;
file_count++;
}
}
closedir(dir);
return file_count;
}
```
这个函数接受一个文件夹路径和一个保存文件信息的结构体数组,返回文件数量。它会遍历文件夹内的所有文件,如果是普通文件,就将文件名保存到结构体中,并将数量初始化为0。
3. 编写一个函数来获取每个文件的数量:
```
void count_file(char *folder_path, FileInfo *file_info, int file_count) {
DIR *dir;
struct dirent *entry;
struct stat statbuf;
char path[256];
for (int i = 0; i < file_count; i++) {
int count = 0;
sprintf(path, "%s/%s", folder_path, file_info[i].filename);
dir = opendir(path);
if (dir == NULL) {
perror("opendir error");
return;
}
while ((entry = readdir(dir)) != NULL) {
if (entry->d_type == DT_REG) {
char file_path[256];
sprintf(file_path, "%s/%s", path, entry->d_name);
if (stat(file_path, &statbuf) == 0) {
count++;
}
}
}
closedir(dir);
file_info[i].count = count;
}
}
```
这个函数接受文件夹路径、文件信息结构体数组和文件数量作为参数。它会遍历每个文件夹,统计文件数量,并将数量保存到对应的结构体中。
4. 主函数中调用以上两个函数:
```
int main() {
char folder_path[256] = "/path/to/folder";
FileInfo file_info[100];
int file_count = get_file_info(folder_path, file_info);
count_file(folder_path, file_info, file_count);
for (int i = 0; i < file_count; i++) {
printf("filename: %s, count: %d\n", file_info[i].filename, file_info[i].count);
}
return 0;
}
```
这个示例代码中的`folder_path`可以替换成你要处理的日期文件夹的路径。运行程序后,它会输出每个文件的名字和数量。
阅读全文
相关推荐
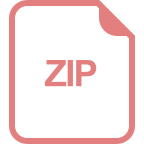
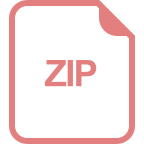
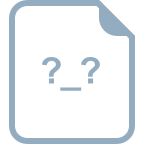
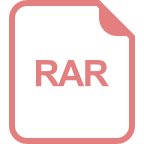
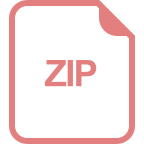
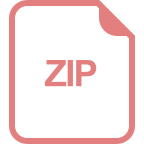
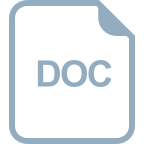
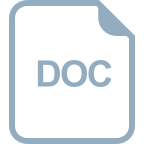
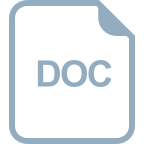
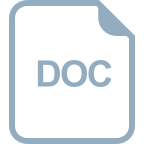
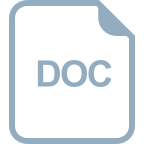
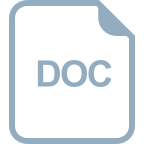
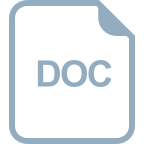
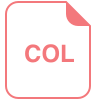
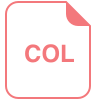
