用c语言编译已知元素x在顺序表上的插入位置(序号i),编写程序完成在顺序表上的插入功能,将编写好的函数在主函数的调用。 bool ListInsert(SqList *&L,int i,ElemType e){ }
时间: 2024-10-16 21:22:29 浏览: 25
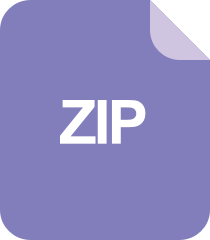
C语言 使用自定义顺序表实现非递减顺序表合并
在C语言中,为了在顺序列表(SqList)上插入元素e,首先需要确保有正确的数据结构定义,如链表的节点包含一个值域(ElemType)和指向下一个节点的指针。对于给定的已知元素x和索引i,我们可以创建一个新的节点并将其添加到现有列表的适当位置。这里是一个简单的示例:
```c
#include <stdbool.h>
// 定义顺序列表节点结构体
typedef struct SqListNode {
ElemType data; // 节点的数据域
struct SqListNode* next; // 指向下一个节点的指针
} SqListNode;
// 插入函数原型
bool ListInsert(SqList *&L, int i, const ElemType e) {
// 如果输入的索引超出范围(小于0或大于链表长度)
if (i <= 0 || L == NULL || i > ListLength(L)) {
return false;
}
// 创建新节点
SqListNode* newNode = (SqListNode*)malloc(sizeof(SqListNode));
newNode->data = e;
newNode->next = NULL;
// 如果插入位置是链表的第一个元素
if (i == 1) {
newNode->next = L;
L = newNode;
}
else {
SqListNode *current = L;
for (int j = 1; j < i; ++j) {
current = current->next;
}
newNode->next = current->next;
current->next = newNode;
}
return true; // 成功插入,返回true
}
// 判断链表是否为空的辅助函数
size_t ListLength(const SqList *L) {
size_t length = 0;
while (L != NULL) {
++length;
L = L->next;
}
return length;
}
// 主函数调用示例
int main() {
SqList *list = NULL; // 初始化空链表
// 具体使用场景:假设我们有一个元素x和索引i
const ElemType x = 5;
int i = 3;
if (ListInsert(&list, i, x)) {
printf("Element inserted at position %d successfully.\n", i);
} else {
printf("Failed to insert element at position %d.\n", i);
}
// ...其他操作,例如释放内存等...
return 0;
}
```
在这个例子中,`ListInsert`函数会检查索引的有效性,并根据索引将新元素插入到正确的位置。注意,你需要确保在主函数结束后适当地管理动态分配的内存。
阅读全文
相关推荐
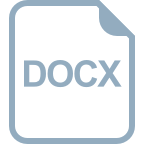
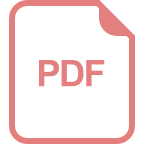
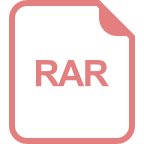

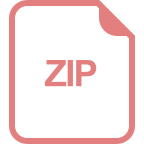
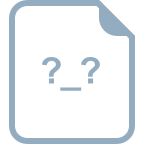
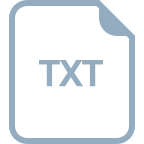
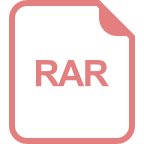
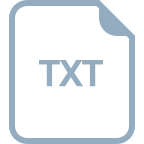
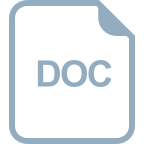
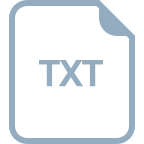
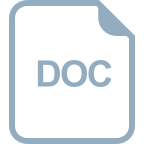
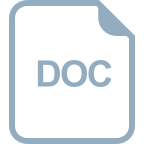
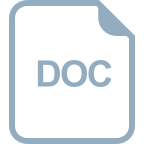