使用tcpdump命令获取网络包 C/C++
时间: 2023-08-03 17:05:21 浏览: 239
可以使用libpcap库来编写C/C++程序获取网络包。下面是一个简单的示例代码,可以获取指定网卡的所有TCP包:
```c++
#include <stdio.h>
#include <pcap.h>
#include <netinet/in.h>
#include <netinet/tcp.h>
void got_packet(u_char *args, const struct pcap_pkthdr *header, const u_char *packet) {
struct ether_header *eth = (struct ether_header *)packet;
if (ntohs(eth->ether_type) == ETHERTYPE_IP) {
struct iphdr *iph = (struct iphdr *)(packet + sizeof(struct ether_header));
if (iph->protocol == IPPROTO_TCP) {
struct tcphdr *tcph = (struct tcphdr *)(packet + sizeof(struct ether_header) + sizeof(struct iphdr));
printf("TCP packet: %s:%d -> %s:%d\n", inet_ntoa(*(in_addr *)&iph->saddr), ntohs(tcph->source), inet_ntoa(*(in_addr *)&iph->daddr), ntohs(tcph->dest));
}
}
}
int main(int argc, char **argv) {
char errbuf[PCAP_ERRBUF_SIZE];
pcap_t *handle = pcap_open_live(argv[1], BUFSIZ, 1, 1000, errbuf);
if (!handle) {
printf("pcap_open_live() failed: %s\n", errbuf);
return 1;
}
if (pcap_datalink(handle) != DLT_EN10MB) {
printf("Device not Ethernet\n");
return 1;
}
if (pcap_setfilter(handle, pcap_compile(handle, "tcp", 1, 0)) != 0) {
printf("pcap_setfilter() failed\n");
return 1;
}
printf("Listening on %s...\n", argv[1]);
pcap_loop(handle, -1, got_packet, NULL);
pcap_close(handle);
return 0;
}
```
使用方法:
```bash
$ sudo ./get_tcp_packets eth0
```
其中,eth0是你要监听的网卡名称。注意需要以root权限运行。
阅读全文
相关推荐
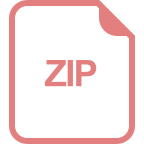
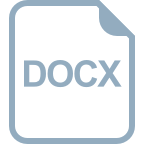

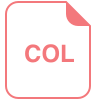
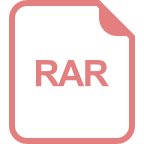
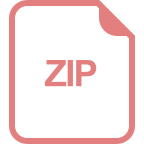
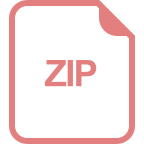
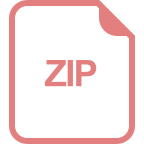
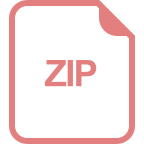
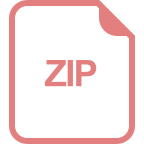
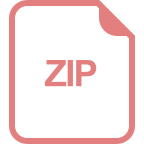
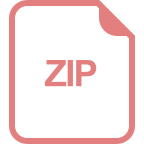