raw_weights = torch.bmm(x, x.transpose(1, 2)) weights = F.softmax(raw_weights, dim=2)
时间: 2024-06-03 16:08:54 浏览: 155
These two lines of code are performing a matrix multiplication between the tensor x and its transpose, followed by a softmax operation along the last dimension.
The resulting tensor, weights, represents a probability distribution over the dimensions of the input tensor x. This can be interpreted as a set of weights that determine the importance of each input dimension in the output of subsequent operations.
This operation is commonly used in neural network architectures such as attention mechanisms, where the weights are used to focus on certain parts of the input during computation.
相关问题
用python复现torch.nn.MultiheadAttention中参数key_padding_mask的功能
要复现`torch.nn.MultiheadAttention`中`key_padding_mask`参数的功能,你可以使用torch.masked_fill函数。以下是一个示例代码:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class MultiheadAttention(nn.Module):
def __init__(self, embed_dim, num_heads):
super(MultiheadAttention, self).__init__()
self.embed_dim = embed_dim
self.num_heads = num_heads
# 线性变换层
self.q_linear = nn.Linear(embed_dim, embed_dim)
self.k_linear = nn.Linear(embed_dim, embed_dim)
self.v_linear = nn.Linear(embed_dim, embed_dim)
# 输出线性层
self.out_linear = nn.Linear(embed_dim, embed_dim)
def forward(self, query, key, value, key_padding_mask=None):
batch_size = query.size(0)
seq_len = query.size(1)
# 线性变换
query = self.q_linear(query)
key = self.k_linear(key)
value = self.v_linear(value)
# 改变形状以便多头注意力计算
query = query.view(batch_size * self.num_heads, seq_len, self.embed_dim // self.num_heads)
key = key.view(batch_size * self.num_heads, -1, self.embed_dim // self.num_heads)
value = value.view(batch_size * self.num_heads, -1, self.embed_dim // self.num_heads)
# 计算注意力得分
scores = torch.bmm(query, key.transpose(1, 2))
if key_padding_mask is not None:
key_padding_mask = key_padding_mask.unsqueeze(1).unsqueeze(2)
scores = scores.masked_fill(key_padding_mask, float('-inf'))
# 注意力权重归一化
attn_weights = F.softmax(scores, dim=-1)
# 加权求和
attn_output = torch.bmm(attn_weights, value)
# 恢复形状
attn_output = attn_output.view(batch_size, seq_len, self.embed_dim)
# 输出线性变换
attn_output = self.out_linear(attn_output)
return attn_output
```
在上述代码中,我们在`forward`方法中添加了一个名为`key_padding_mask`的参数。在计算注意力得分之前,我们将`key_padding_mask`进行扩展和形状调整,以使其与注意力得分的形状相匹配。然后,我们使用torch.masked_fill函数将`key_padding_mask`中为True的位置对应的注意力得分设置为负无穷(-inf),以进行屏蔽。最后,我们继续进行注意力权重归一化、加权求和等操作。
请注意,这个实现是一个简化版本,并没有包含所有的细节和优化。如果需要更完整和高效的实现,可以参考PyTorch官方文档或其他相关资源。
用python复现torch.nn.MultiheadAttention的功能
要复现`torch.nn.MultiheadAttention`的功能,你需要进行一些额外的准备工作,并编写一些自定义代码。以下是一个简化版本的示例代码:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class MultiheadAttention(nn.Module):
def __init__(self, embed_dim, num_heads):
super(MultiheadAttention, self).__init__()
self.embed_dim = embed_dim
self.num_heads = num_heads
# 线性变换层
self.q_linear = nn.Linear(embed_dim, embed_dim)
self.k_linear = nn.Linear(embed_dim, embed_dim)
self.v_linear = nn.Linear(embed_dim, embed_dim)
# 输出线性层
self.out_linear = nn.Linear(embed_dim, embed_dim)
def forward(self, query, key, value, attn_mask=None):
batch_size = query.size(0)
# 线性变换
query = self.q_linear(query)
key = self.k_linear(key)
value = self.v_linear(value)
# 改变形状以便多头注意力计算
query = query.view(batch_size * self.num_heads, -1, self.embed_dim // self.num_heads)
key = key.view(batch_size * self.num_heads, -1, self.embed_dim // self.num_heads)
value = value.view(batch_size * self.num_heads, -1, self.embed_dim // self.num_heads)
# 计算注意力得分
scores = torch.bmm(query, key.transpose(1, 2))
if attn_mask is not None:
scores = scores.masked_fill(attn_mask.unsqueeze(1), float('-inf'))
# 注意力权重归一化
attn_weights = F.softmax(scores, dim=-1)
# 加权求和
attn_output = torch.bmm(attn_weights, value)
# 恢复形状
attn_output = attn_output.view(batch_size, -1, self.embed_dim)
# 输出线性变换
attn_output = self.out_linear(attn_output)
return attn_output
```
在上述代码中,我们首先定义了一个名为`MultiheadAttention`的自定义模块。在`__init__`方法中,我们初始化了线性变换层和输出线性层。在`forward`方法中,我们首先对查询(query)、键(key)和值(value)进行线性变换,然后将形状调整为适合多头注意力计算的形式。接下来,我们计算注意力得分,并根据给定的注意力掩码进行填充。然后,我们对注意力权重进行归一化,并将其与值进行加权求和。最后,我们恢复形状,并对输出进行线性变换。请注意,这个实现是一个简化版本,并没有包含所有的细节和优化。如果需要更完整和高效的实现,可以参考PyTorch官方文档或其他相关资源。
阅读全文
相关推荐
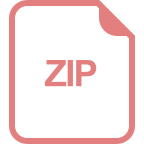
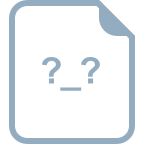
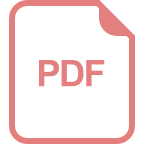
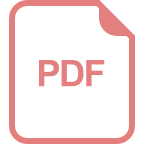









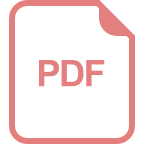
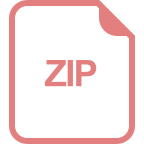
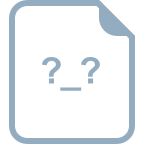