用java实现图形化进程调度代码RR可运行
时间: 2023-09-30 10:10:42 浏览: 88
以下是使用 Java 实现的轮转调度算法(RR)的图形化进程调度代码,供参考:
```java
import java.awt.Color;
import java.awt.Font;
import java.awt.Graphics;
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.Queue;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class RR extends JPanel {
private static final long serialVersionUID = 1L;
private static final int WIDTH = 800;
private static final int HEIGHT = 600;
private static final int TIME_QUANTUM = 20; // 时间片
private static final int ROW_HEIGHT = 50;
private static final int ROW_WIDTH = 100;
private static final int GAP = 10;
private static final int FONT_SIZE = 16;
private static final int PROCESS_COUNT = 5; // 进程数
private static final Color[] COLORS = { Color.RED, Color.BLUE, Color.GREEN, Color.ORANGE, Color.MAGENTA };
private static final String[] PROCESS_NAMES = { "P1", "P2", "P3", "P4", "P5" };
private static final int[] BURST_TIMES = { 80, 50, 120, 65, 90 }; // 进程的 CPU 执行时间
private static final int[] ARRIVAL_TIMES = { 0, 20, 30, 40, 60 }; // 进程到达时间
private static int time = 0; // 运行时间
private static int currentProcess = -1; // 当前运行的进程
private static int currentBurstTime = 0; // 当前进程剩余的 CPU 执行时间
private static Queue<Integer> readyQueue = new LinkedList<>(); // 就绪队列
private static ArrayList<Process> processes = new ArrayList<>(); // 进程列表
public RR() {
JFrame frame = new JFrame("Round Robin Scheduling");
frame.setSize(WIDTH, HEIGHT);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(this);
frame.setVisible(true);
// 初始化进程列表
for (int i = 0; i < PROCESS_COUNT; i++) {
processes.add(new Process(PROCESS_NAMES[i], BURST_TIMES[i], ARRIVAL_TIMES[i], COLORS[i]));
}
while (true) {
repaint();
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
// 处理到达的进程
for (int i = 0; i < PROCESS_COUNT; i++) {
if (processes.get(i).arrivalTime == time) {
readyQueue.offer(i);
}
}
// 如果当前没有进程在执行,则从就绪队列中取出一个进程开始执行
if (currentProcess == -1 && !readyQueue.isEmpty()) {
currentProcess = readyQueue.poll();
currentBurstTime = processes.get(currentProcess).burstTime;
}
// 执行当前进程
if (currentProcess != -1) {
currentBurstTime -= TIME_QUANTUM;
processes.get(currentProcess).remainingTime -= TIME_QUANTUM;
if (currentBurstTime <= 0) {
currentProcess = -1;
currentBurstTime = 0;
processes.get(currentProcess).completeTime = time;
} else if (processes.get(currentProcess).remainingTime <= 0) {
currentProcess = -1;
currentBurstTime = 0;
processes.get(currentProcess).completeTime = time;
} else {
readyQueue.offer(currentProcess);
currentProcess = readyQueue.poll();
currentBurstTime = Math.min(processes.get(currentProcess).remainingTime, TIME_QUANTUM);
}
}
time += TIME_QUANTUM;
// 如果所有进程都已完成,则退出循环
boolean allCompleted = true;
for (int i = 0; i < PROCESS_COUNT; i++) {
if (!processes.get(i).isCompleted()) {
allCompleted = false;
break;
}
}
if (allCompleted) {
break;
}
}
}
@Override
public void paint(Graphics g) {
super.paint(g);
// 绘制时间轴
g.setColor(Color.BLACK);
g.drawLine(0, HEIGHT - ROW_HEIGHT - GAP, WIDTH, HEIGHT - ROW_HEIGHT - GAP);
for (int i = 0; i <= time; i += 20) {
int x = i * ROW_WIDTH / 20;
g.drawLine(x, HEIGHT - ROW_HEIGHT - GAP, x, HEIGHT - ROW_HEIGHT - GAP + GAP / 2);
g.drawString(Integer.toString(i), x - 5, HEIGHT - ROW_HEIGHT - GAP / 2);
}
// 绘制进程
for (int i = 0; i < PROCESS_COUNT; i++) {
Process p = processes.get(i);
g.setColor(p.color);
g.fillRect(p.arrivalTime * ROW_WIDTH / 20, i * ROW_HEIGHT, p.completeTime * ROW_WIDTH / 20 - p.arrivalTime * ROW_WIDTH / 20, ROW_HEIGHT);
g.setColor(Color.BLACK);
g.drawRect(p.arrivalTime * ROW_WIDTH / 20, i * ROW_HEIGHT, p.completeTime * ROW_WIDTH / 20 - p.arrivalTime * ROW_WIDTH / 20, ROW_HEIGHT);
g.setFont(new Font("Arial", Font.PLAIN, FONT_SIZE));
g.drawString(p.name, p.arrivalTime * ROW_WIDTH / 20 + 5, i * ROW_HEIGHT + ROW_HEIGHT / 2 + FONT_SIZE / 2);
}
// 绘制当前进程
if (currentProcess != -1) {
Process p = processes.get(currentProcess);
g.setColor(p.color);
g.fillRect(p.arrivalTime * ROW_WIDTH / 20, currentProcess * ROW_HEIGHT, (time - p.arrivalTime) * ROW_WIDTH / 20 - GAP, ROW_HEIGHT);
g.setColor(Color.BLACK);
g.drawRect(p.arrivalTime * ROW_WIDTH / 20, currentProcess * ROW_HEIGHT, (time - p.arrivalTime) * ROW_WIDTH / 20 - GAP, ROW_HEIGHT);
g.setFont(new Font("Arial", Font.PLAIN, FONT_SIZE));
g.setColor(Color.WHITE);
g.drawString(p.name, p.arrivalTime * ROW_WIDTH / 20 + 5, currentProcess * ROW_HEIGHT + ROW_HEIGHT / 2 + FONT_SIZE / 2);
}
}
private static class Process {
public String name;
public int burstTime;
public int arrivalTime;
public int remainingTime;
public int completeTime;
public Color color;
public Process(String name, int burstTime, int arrivalTime, Color color) {
this.name = name;
this.burstTime = burstTime;
this.arrivalTime = arrivalTime;
this.remainingTime = burstTime;
this.color = color;
}
public boolean isCompleted() {
return remainingTime <= 0;
}
}
public static void main(String[] args) {
new RR();
}
}
```
该代码使用 Java Swing 绘制图形界面,实现了轮转调度算法(RR)的进程调度过程,并在界面上展示了每个进程的执行情况。在 `main` 方法中创建了一个 `RR` 对象,即可运行该程序。
阅读全文
相关推荐




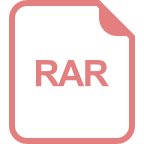



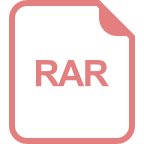







