将上面的代码逐行进行详细的注释
时间: 2024-06-09 14:07:14 浏览: 227
好的,以下是逐行的注释:
```python
import openai
import requests
import json
import os
from dotenv import load_dotenv
```
首先我们导入了需要使用的库,`openai` 用于调用 OpenAI 的 API,`requests` 用于发送 HTTP 请求,`json` 用于处理返回的 JSON 数据,`os` 用于获取环境变量,`dotenv` 用于加载 `.env` 文件中的环境变量。
```python
load_dotenv()
openai.api_key = os.getenv("OPENAI_API_KEY")
```
接着,我们通过 `load_dotenv()` 加载 `.env` 文件中的环境变量,然后使用 `os.getenv()` 获取 OpenAI API Key 并将其赋值给 `openai.api_key`。
```python
def generate_text(prompt):
response = openai.Completion.create(
engine="davinci",
prompt=prompt,
max_tokens=1024,
n=1,
stop=None,
temperature=0.5,
)
message = response.choices[0].text.strip()
return message
```
这个函数用于调用 OpenAI API 生成 AI 的回复。`openai.Completion.create()` 是 OpenAI API 提供的生成文本的方法,这里我们传入了以下参数:
- `engine`:使用的 AI 模型,这里使用的是 `davinci`,是 OpenAI 最强大的模型之一。
- `prompt`:AI 的输入,即我们需要 AI 回复的内容。
- `max_tokens`:AI 输出的最大长度,这里设置为 1024。
- `n`:生成几个回复,这里我们只需要一个回复,所以设置为 1。
- `stop`:用于停止生成的标记,这里设置为 `None`,即不使用任何标记。
- `temperature`:用于控制生成的多样性,这里设置为 0.5。
然后我们从 `response` 中取出 AI 生成的回复,并将其返回。
```python
def send_message(message):
url = "https://api.telegram.org/bot{}/sendMessage".format(TELEGRAM_BOT_TOKEN)
data = {
"chat_id": TELEGRAM_CHAT_ID,
"text": message,
}
response = requests.post(url, data=data)
return response.json()
```
这个函数用于向 Telegram 发送消息。我们向 Telegram 发送消息需要使用 Telegram Bot API,这里我们调用了 `requests.post()` 方法向 Telegram Bot API 发送 POST 请求,请求的 URL 为 `https://api.telegram.org/bot{}/sendMessage`,其中 `{}` 部分需要填写 Telegram Bot 的 token。我们还需要传入以下参数:
- `chat_id`:接收消息的 chat ID,即我们要将消息发送到哪个 chat。
- `text`:消息内容。
然后我们将响应的 JSON 数据返回。
```python
def main():
update_id = None
while True:
updates = get_updates(update_id)
if updates["result"]:
for update in updates["result"]:
update_id = update["update_id"]
try:
message = update["message"]["text"]
chat_id = update["message"]["chat"]["id"]
send_message(generate_text(message))
except Exception as e:
print(e)
```
这个函数是我们的主函数,用于不断地接收来自 Telegram 的消息并生成 AI 回复。首先我们设置了一个变量 `update_id`,用于记录已经接收的最后一条消息的 ID。然后我们进入一个无限循环,不停地调用 `get_updates()` 方法获取最新的消息。如果有新的消息,我们就遍历这些消息并将其处理。对于每个消息,我们获取其文本内容和 chat ID,然后调用 `send_message()` 方法向该 chat 发送 AI 回复。如果在这个过程中出现了异常,我们将异常信息打印出来。
```python
def get_updates(offset=None):
url = "https://api.telegram.org/bot{}/getUpdates".format(TELEGRAM_BOT_TOKEN)
if offset:
url += "?offset={}".format(offset)
response = requests.get(url)
return json.loads(response.content.decode("utf8"))
```
这个函数用于获取 Telegram 的消息。我们向 Telegram 发送 GET 请求,请求的 URL 为 `https://api.telegram.org/bot{}/getUpdates`,其中 `{}` 部分需要填写 Telegram Bot 的 token。如果传入了 `offset`,我们还需要在 URL 中加上 `offset` 参数。然后我们将响应的 JSON 数据解码并返回。
最后,我们调用 `main()` 函数启动程序。
阅读全文
相关推荐
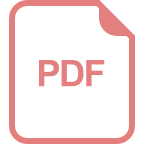
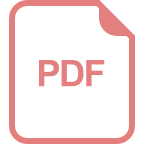
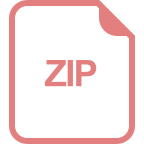
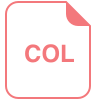
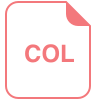
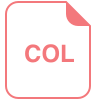
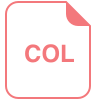
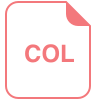
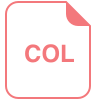
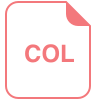
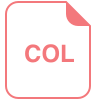





