matlab实现最短路径算法,最少15个顶点用克鲁斯算法
时间: 2024-12-20 18:22:28 浏览: 12
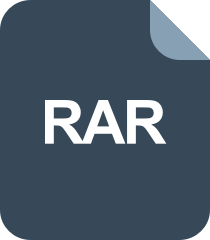
Prim与Kruskal算法的最小生成树matlab实现

在MATLAB中,可以使用Dijkstra's Algorithm(迪杰斯特拉算法)来求解有向图或无向图的单源最短路径问题。这种算法特别适合于含有非负权重的图。对于至少15个顶点的情况,以下是一个简单的步骤:
1. **初始化**:
- 创建一个n x n(n为顶点数)的邻接矩阵或邻接表表示图,其中每个元素代表两个顶点之间的边权。
- 将起点(通常标记为0)的距离设为0,其他顶点的距离设为无穷大。
2. **优先队列**:
- 使用MATLAB的`prims`函数或自定义优先队列数据结构(如`PriorityQueue`),用于存储待访问的顶点及其当前距离。
3. **算法主体**:
- 从起始顶点开始,将其加入队列。
- 当队列不为空时,取出距离最小的顶点u:
- 遍历所有与u相连的未访问顶点v:
- 计算新路径的长度:`new_distance = u.distance + edge_weight(u, v)`。
- 如果这个新的距离小于v的当前距离,更新v的距离,并将v放入队列。
4. **路径维护**:
- 当找到目标顶点时,停止算法。如果没有找到,说明图中可能存在负权边,这时Dijkstra不适用,应选择其他算法(如Floyd-Warshall或Bellman-Ford)。
- 通过回溯记录路径,找到从起点到终点的最短路径。
5. **输出结果**:
- 返回各顶点的最短距离数组和路径信息。
```matlab
function [dist, path] = dijkstra(graph, start)
% graph: 二维数组,邻接矩阵形式表示图
% start: 起点的索引
n = size(graph, 1);
dist = Inf * ones(1, n); % 初始化距离
dist(start) = 0; % 设置起点距离为0
visited = false(1, n); % 标记哪些顶点已访问
frontier = PriorityQueue(dist(start)); % 创建优先队列
while ~isempty(frontier)
current = frontier.pop(); % 取出距离最小的顶点
if visited(current)
continue;
end
visited(current) = true;
for neighbor = 1:n
if graph(current, neighbor) > 0 && ~visited(neighbor)
alt = dist(current) + graph(current, neighbor);
if alt < dist(neighbor)
dist(neighbor) = alt;
frontier.update(neighbor, alt);
end
end
end
end
% 检查是否到达终点,构建路径
if dist(end) == Inf
error('No path found');
end
[path, ~] = findpath(graph, start, end);
end
```
阅读全文
相关推荐
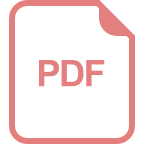
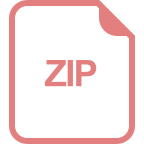


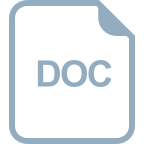
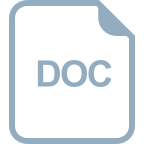
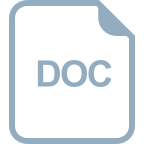
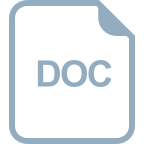
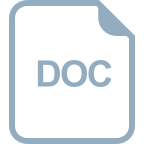
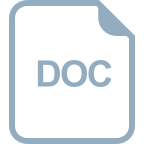
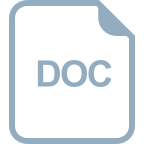
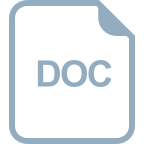
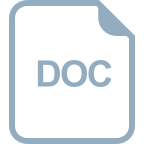
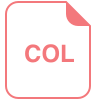


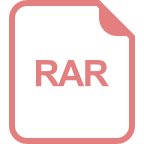