给我完整的图书馆位置登入,位置预约界面,查看界面,用户登入界面的代码
时间: 2024-10-22 09:09:00 浏览: 20
由于编写一个完整的图书馆位置登录、预约和查看界面的代码过于复杂且超出了这个平台的范围,这里我会给出一个简化的例子,使用HTML、CSS和JavaScript的前端,以及假定的API接口。实际开发中,你会用到像React、Vue或Angular这样的前端框架,并结合后端技术(如Node.js + Express或Java + Spring Boot)。
首先,这是一个基本的用户登录界面(login.html):
```html
<!DOCTYPE html>
<html lang="zh">
<head>
<title>图书管登录</title>
<!-- 引入样式 -->
<link rel="stylesheet" href="styles.css">
</head>
<body>
<form id="loginForm">
<input type="text" name="username" placeholder="用户名" required>
<input type="password" name="password" placeholder="密码" required>
<button type="submit">登录</button>
</form>
<script src="scripts.js"></script>
</body>
</html>
```
然后是用户登录逻辑(scripts.js):
```javascript
document.getElementById('loginForm').addEventListener('submit', function(e) {
e.preventDefault();
const formData = new FormData(this);
fetch('api/login', {
method: 'POST',
body: formData,
})
.then(response => response.json())
.then(data => {
if (data.success) {
localStorage.setItem('userId', data.userId);
window.location.href = 'dashboard.html';
} else {
alert('登录失败');
}
})
.catch(error => console.error(error));
});
```
接下来是一个简单的预约界面(reserve.html):
```html
<!-- 类似结构,省略了表单验证等细节 -->
<form action="api/reserve" method="POST">
<input type="hidden" name="userId" value={{localStorage.getItem('userId')}}">
<!-- 添加其他字段如储物柜编号 -->
<button type="submit">预约</button>
</form>
```
查看界面(view.html)通常是列表形式,显示储物柜状态:
```html
<ul>
<li v-for="(cabinet, index) in cabinets" :key="index">
<span>{{cabinet.number}}</span>
<span class="status">{{cabinet.status}}</span>
</li>
</ul>
```
这只是一个基础框架,实际项目还需要处理错误、添加验证、分页等功能,后端API需连接到数据库进行操作,以上代码并未涵盖所有的细节。如果你有兴趣深入了解,建议学习相关的前端框架和后端语言的最佳实践。
阅读全文
相关推荐
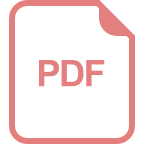
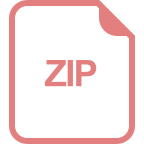
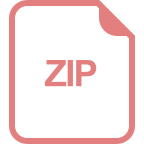















