export const downloadFile = (data,fileName)=>{ if (!data) { return } let url = window.URL.createObjectURL(new Blob([data])) let link = document.createElement('a') link.style.display = 'none' link.href = url link.setAttribute('download', `${fileName}`) document.body.appendChild(link) link.click() } 解释一下这段代码
时间: 2024-04-18 20:27:45 浏览: 10
这段代码是一个函数 `downloadFile`,用于下载文件。它接受两个参数:`data`表示文件的内容,`fileName`表示文件的名称。
首先,它会检查 `data` 是否存在,如果不存在则直接返回,不进行下载操作。
接下来,它会创建一个 URL 对象,该对象将 `data` 封装成一个 Blob 对象,并生成一个可用于访问的 URL。
然后,它创建一个 `<a>` 标签元素,设置其样式为 `display: none`(不显示在页面上),将之前生成的 URL 设置为其 `href` 属性值。同时,通过 `setAttribute` 方法设置 `download` 属性为传入的 `fileName`。
接着,它将这个 `<a>` 标签添加到页面的 `<body>` 元素中。
最后,通过调用 `link.click()` 方法,模拟点击下载链接,从而触发浏览器下载该文件。
总结来说,这段代码的作用是通过 Blob 对象生成文件下载链接,并模拟点击该链接实现文件的下载。
相关问题
请使用Pointfree 编程风格改造以下代码:export const getObjectURL = (file) => { let url = null; if (window.createObjectURL !== undefined) { // basic url = window.createObjectURL(file); } else if (window.webkitURL !== undefined) { // webkit or chrome try { url = window.webkitURL.createObjectURL(file); } catch (error) { console.log(error); } } else if (window.URL !== undefined) { // mozilla(firefox) try { url = window.URL.createObjectURL(file); } catch (error) { console.log(error); } } return url; }
非常感谢您的提问!我理解您想让我使用 Pointfree 编程风格重构 getObjectURL 函数。下面是重构后的代码:
export const getObjectURL = (file) => {
const objectURL = (window.createObjectURL || window.webkitURL.createObjectURL || window.URL.createObjectURL)
try {
return objectURL(file)
} catch (error) {
console.log(error)
return null
}
}
在这个重构后的代码中,我们使用了函数式编程中的 Pointfree 风格。将需要操作的数据 file 和函数实现分离出来,使得代码逻辑更加清晰。我们使用了柯里化的方式,将后面的 window.createObjectURL 等三种函数封装成一个函数 objectURL,避免了 if-else 的使用,提高代码执行效率。除此之外,我们还使用了 try-catch 语句,捕获运行时可能出现的错误,确保代码的稳定性和健壮性。
希望这个回答能够帮助您,如果您还有其它问题,请随时提出哦!
请使用函数式编程,改造以下代码, export const getObjectURL = (file) => { let url = null; if (window.createObjectURL !== undefined) { // basic url = window.createObjectURL(file); } else if (window.webkitURL !== undefined) { // webkit or chrome try { url = window.webkitURL.createObjectURL(file); } catch (error) { console.log(error); } } else if (window.URL !== undefined) { // mozilla(firefox) try { url = window.URL.createObjectURL(file); } catch (error) { console.log(error); } } return url; }
const getObjectURL = (file) => {
const createObjectURL = window.createObjectURL || window.webkitURL.createObjectURL || window.URL.createObjectURL;
try {
return createObjectURL(file);
} catch (error) {
console.log(error);
return null;
}
}
使用函数式编程重构 getObjectURL() 函数,通过使用箭头函数和 const 关键字来定义一个新的函数。我们将之前的代码抽象成一个 createObjectURL 的变量,然后使用 try/catch 结构来处理异常情况。最后函数返回新的 url 值或者返回 null。
相关推荐
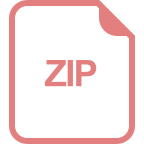
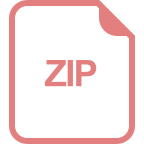
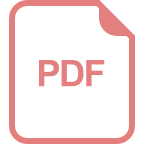













